c++实现复数的加减乘除
时间: 2023-08-23 10:16:29 浏览: 54
以下是C++代码实现复数的加减乘除:
```cpp
#include <iostream>
using namespace std;
class Complex {
private:
double real;
double imag;
public:
Complex(double r = 0, double i = 0) :real(r), imag(i) {}
Complex operator +(const Complex& c2) const {
return Complex(real + c2.real, imag + c2.imag);
}
Complex operator -(const Complex& c2) const {
return Complex(real - c2.real, imag - c2.imag);
}
Complex operator *(const Complex& c2) const {
return Complex(real*c2.real - imag*c2.imag, real*c2.imag + imag*c2.real);
}
Complex operator /(const Complex& c2) const {
double denominator = c2.real*c2.real + c2.imag*c2.imag;
return Complex((real*c2.real + imag*c2.imag) / denominator, (imag*c2.real - real*c2.imag) / denominator);
}
friend ostream& operator<< (ostream& os, const Complex& c);
};
ostream& operator<< (ostream& os, const Complex& c) {
os << c.real << "+" << c.imag << "i";
return os;
}
int main() {
Complex c1(1, 2);
Complex c2(2, 3);
cout << "c1 = " << c1 << endl;
cout << "c2 = " << c2 << endl;
cout << "c1 + c2 = " << c1 + c2 << endl;
cout << "c1 - c2 = " << c1 - c2 << endl;
cout << "c1 * c2 = " << c1 * c2 << endl;
cout << "c1 / c2 = " << c1 / c2 << endl;
return 0;
}
```
其中,重载运算符 `+`、`-`、`*`、`/` 分别表示复数的加、减、乘、除。注意在除法运算中需要对分母做非零判断,以避免除数为零的情况。此外,为了方便输出复数,还重载了输出运算符 `<<`。
相关推荐
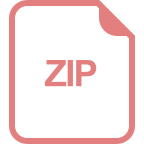














