重载运算符“+”“-”“*”“/”“<<”“>>”,使其能够实现负数的直接输入输出,并实现复数的加减乘除基本运算
时间: 2023-10-02 22:05:18 浏览: 68
好的,这是一个关于重载运算符的问题,我会尽力回答。首先需要明确的是,重载运算符是一种 C++ 中的特殊函数,它可以被用来定义类的基本运算符的行为。由于我们需要实现复数的基本运算,所以我们需要定义一个复数类,并重载运算符“+”“-”“*”“/”“<<”“>>”来实现基本运算。
对于负数的直接输入输出,我们可以通过重载“<<”和“>>”运算符来实现。我们可以定义一个输入输出流,然后重载运算符,使其能够直接输入输出负数。
接下来,我们需要定义一个复数类,其中包含实部和虚部两个成员变量。然后,我们可以重载“+”、“-”、“*”和“/”运算符,使其能够实现复数的加减乘除基本运算。
下面是一个简单的实现代码示例:
```c++
#include <iostream>
using namespace std;
class Complex {
public:
Complex(double r = 0.0, double i = 0.0) : real(r), imag(i) {}
Complex operator+(const Complex &c2) const; // 重载加法运算符
Complex operator-(const Complex &c2) const; // 重载减法运算符
Complex operator*(const Complex &c2) const; // 重载乘法运算符
Complex operator/(const Complex &c2) const; // 重载除法运算符
friend ostream &operator<<(ostream &out, const Complex &c); // 重载输出运算符
friend istream &operator>>(istream &in, Complex &c); // 重载输入运算符
private:
double real;
double imag;
};
Complex Complex::operator+(const Complex &c2) const {
return Complex(real + c2.real, imag + c2.imag);
}
Complex Complex::operator-(const Complex &c2) const {
return Complex(real - c2.real, imag - c2.imag);
}
Complex Complex::operator*(const Complex &c2) const {
return Complex(real * c2.real - imag * c2.imag, real * c2.imag + imag * c2.real);
}
Complex Complex::operator/(const Complex &c2) const {
double r = c2.real * c2.real + c2.imag * c2.imag;
return Complex((real * c2.real + imag * c2.imag) / r, (imag * c2.real - real * c2.imag) / r);
}
ostream &operator<<(ostream &out, const Complex &c) {
out << c.real << "+" << c.imag << "i";
return out;
}
istream &operator>>(istream &in, Complex &c) {
in >> c.real >> c.imag;
return in;
}
int main() {
Complex c1, c2;
cin >> c1 >> c2;
cout << "c1 + c2 = " << c1 + c2 << endl;
cout << "c1 - c2 = " << c1 - c2 << endl;
cout << "c1 * c2 = " << c1 * c2 << endl;
cout << "c1 / c2 = " << c1 / c2 << endl;
return 0;
}
```
在上面的代码中,我们定义了一个名为“Complex”的类,并重载了运算符“+”、“-”、“*”和“/”来实现复数的加减乘除基本运算。同时,我们还重载了输入输出运算符“<<”和“>>”,以支持负数的直接输入输出。
相关推荐
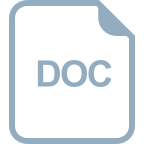
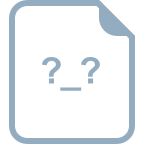















