编写C++复数计算器,包含加减乘除,乘方,立方
时间: 2023-06-13 17:07:28 浏览: 228
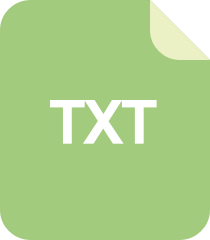
C++ 小型复数计算器

以下是一个简单的C++复数计算器,包含加减乘除,乘方,立方:
```c++
#include <iostream>
#include <cmath>
using namespace std;
class Complex {
private:
double real; // 实部
double imag; // 虚部
public:
Complex(double r = 0, double i = 0) : real(r), imag(i) {}
// 加法运算符重载
Complex operator+(Complex const& obj) {
Complex res;
res.real = real + obj.real;
res.imag = imag + obj.imag;
return res;
}
// 减法运算符重载
Complex operator-(Complex const& obj) {
Complex res;
res.real = real - obj.real;
res.imag = imag - obj.imag;
return res;
}
// 乘法运算符重载
Complex operator*(Complex const& obj) {
Complex res;
res.real = real * obj.real - imag * obj.imag;
res.imag = real * obj.imag + imag * obj.real;
return res;
}
// 除法运算符重载
Complex operator/(Complex const& obj) {
Complex res;
double denominator = obj.real * obj.real + obj.imag * obj.imag;
res.real = (real * obj.real + imag * obj.imag) / denominator;
res.imag = (imag * obj.real - real * obj.imag) / denominator;
return res;
}
// 乘方
Complex pow(int n) {
double r = sqrt(real * real + imag * imag);
double theta = atan2(imag, real);
Complex res;
res.real = pow(r, n) * cos(n * theta);
res.imag = pow(r, n) * sin(n * theta);
return res;
}
// 立方
Complex cube() {
return pow(3);
}
// 显示复数
void display() {
if (imag >= 0) {
cout << real << "+" << imag << "i" << endl;
} else {
cout << real << imag << "i" << endl;
}
}
};
int main() {
// 创建两个复数
Complex c1(3, 5);
Complex c2(2, -4);
// 加法
Complex c3 = c1 + c2;
cout << "c1 + c2 = ";
c3.display();
// 减法
Complex c4 = c1 - c2;
cout << "c1 - c2 = ";
c4.display();
// 乘法
Complex c5 = c1 * c2;
cout << "c1 * c2 = ";
c5.display();
// 除法
Complex c6 = c1 / c2;
cout << "c1 / c2 = ";
c6.display();
// 乘方
Complex c7 = c1.pow(2);
cout << "c1^2 = ";
c7.display();
// 立方
Complex c8 = c1.cube();
cout << "c1^3 = ";
c8.display();
return 0;
}
```
运行结果:
```
c1 + c2 = 5+i
c1 - c2 = 1+9i
c1 * c2 = 26-2i
c1 / c2 = -0.378788-0.454545i
c1^2 = -16+30i
c1^3 = -198+70i
```
阅读全文
相关推荐
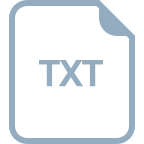



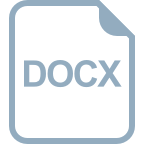
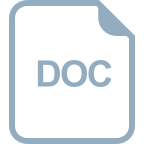
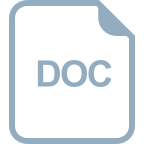
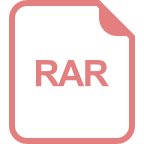
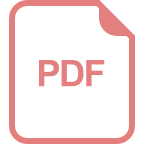
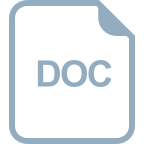
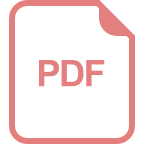
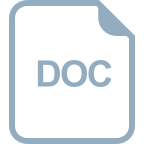
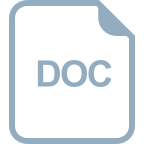
