复数的加减运算(运算符重载)
时间: 2023-06-21 19:22:15 浏览: 240
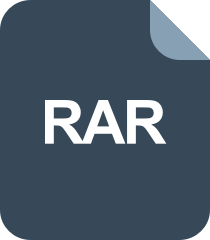
FS_C_复数_www./fs.c.com_
复数的加减运算可以使用运算符重载来实现。针对复数类,我们可以重载加减运算符,使其能够对复数进行加减运算。
具体实现方法如下:
1. 定义一个复数类,包含实部和虚部两个成员变量。
2. 重载加法运算符+,使其能够对两个复数进行加法运算。
3. 重载减法运算符-,使其能够对两个复数进行减法运算。
示例代码如下:
```c++
#include <iostream>
using namespace std;
class Complex
{
public:
Complex(double r=0.0, double i=0.0):real(r), imag(i){}
Complex operator+(const Complex& c2) const; // 加法运算符重载
Complex operator-(const Complex& c2) const; // 减法运算符重载
void display() const; // 显示复数
private:
double real; // 实部
double imag; // 虚部
};
Complex Complex::operator+(const Complex& c2) const
{
return Complex(real+c2.real, imag+c2.imag);
}
Complex Complex::operator-(const Complex& c2) const
{
return Complex(real-c2.real, imag-c2.imag);
}
void Complex::display() const
{
cout << "(" << real << "," << imag << "i)" << endl;
}
int main()
{
Complex c1(1.0, 2.0);
Complex c2(3.0, 4.0);
Complex c3;
c3 = c1 + c2; // 复数加法运算
cout << "c1 + c2 = ";
c3.display();
c3 = c1 - c2; // 复数减法运算
cout << "c1 - c2 = ";
c3.display();
return 0;
}
```
输出结果如下:
```
c1 + c2 = (4,6i)
c1 - c2 = (-2,-2i)
```
以上就是复数加减运算的实现方法。
阅读全文
相关推荐
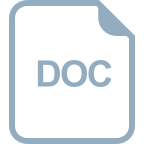
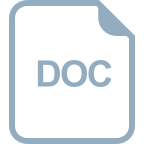

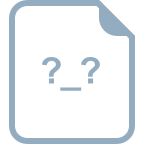
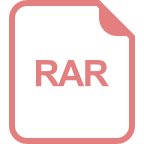
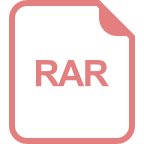
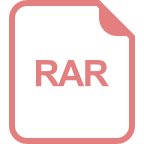
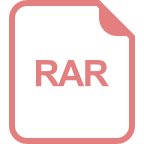
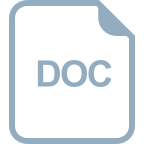
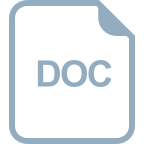




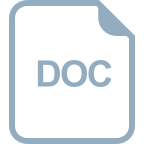
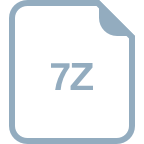
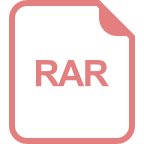