设计一个序列检测器检测序列1110010,每输入一个序列1110010则会在输出端输出一个1,其余时间为0。给出VHDL代码
时间: 2024-02-03 11:13:15 浏览: 177
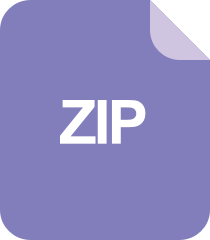
用VHDL设计移位寄存器.zip_VHDL设计寄存器_vhdl_vhdl序列检测

以下是一个基于Moore状态机的VHDL代码,实现了对序列"1110010"的检测器。
```vhdl
library IEEE;
use IEEE.STD_LOGIC_1164.ALL;
use IEEE.STD_LOGIC_UNSIGNED.ALL;
entity sequence_detector is
Port ( clk: in STD_LOGIC;
reset: in STD_LOGIC;
input: in STD_LOGIC;
output: out STD_LOGIC);
end sequence_detector;
architecture Behavioral of sequence_detector is
type state_type is (s0, s1, s2, s3, s4, s5, s6);
signal present_state, next_state: state_type;
begin
-- Moore state machine
process (clk, reset)
begin
if reset = '1' then
present_state <= s0;
elsif rising_edge(clk) then
present_state <= next_state;
end if;
end process;
-- State transitions
process (present_state, input)
begin
case present_state is
when s0 =>
if input = '1' then
next_state <= s1;
else
next_state <= s0;
end if;
when s1 =>
if input = '1' then
next_state <= s2;
else
next_state <= s0;
end if;
when s2 =>
if input = '1' then
next_state <= s3;
else
next_state <= s0;
end if;
when s3 =>
if input = '0' then
next_state <= s4;
else
next_state <= s0;
end if;
when s4 =>
if input = '0' then
next_state <= s5;
else
next_state <= s0;
end if;
when s5 =>
if input = '1' then
next_state <= s6;
else
next_state <= s0;
end if;
when s6 =>
-- Sequence detected, output a 1
output <= '1';
next_state <= s0;
end case;
end process;
end Behavioral;
```
在此代码中,我们定义了一个 `state_type` 枚举类型来表示状态机的所有状态。然后,我们声明了一个名为 `present_state` 的信号来记录当前状态,以及一个名为 `next_state` 的信号来记录下一个状态。在时钟上升沿时,我们将 `present_state` 更新为 `next_state`。
接下来,我们定义了一个 `process` 块,用于实现状态转移逻辑。我们使用 `case` 语句来处理每个状态,并根据输入信号 `input` 的值更新下一个状态 `next_state`。如果检测到序列"1110010",则输出信号 `output` 被设置为 1,并将下一个状态设置为初始状态 `s0`。
最后,我们可以将此代码综合并烧录到目标 FPGA 上,以实现对序列"1110010"的检测器。
阅读全文
相关推荐
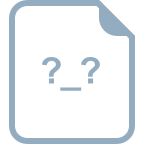



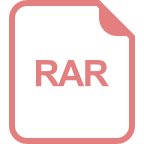
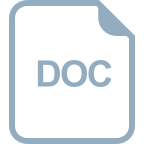
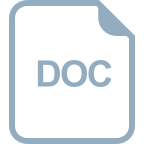
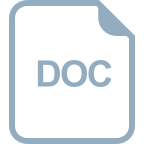
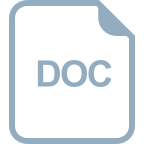
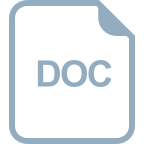






