module bin2bcd(input [4:0] bin, output [7:0] bcd); always @(*) begin case(bin) 5'b00000: bcd = 8'b00000000; 5'b00001: bcd = 8'b00000001; 5'b00010: bcd = 8'b00000010; 5'b00011: bcd = 8'b00000011; 5'b00100: bcd = 8'b00000100; 5'b00101: bcd = 8'b00000101; 5'b00110: bcd = 8'b00000110; 5'b00111: bcd = 8'b00000111; 5'b01000: bcd = 8'b00001000; 5'b01001: bcd = 8'b00001001; 5'b01010: bcd = 8'b00010000; 5'b01011: bcd = 8'b00010001; 5'b01100: bcd = 8'b00010010; 5'b01101: bcd = 8'b00010011; 5'b01110: bcd = 8'b00010100; 5'b01111: bcd = 8'b00010101; 5'b10000: bcd = 8'b00100000; 5'b10001: bcd = 8'b00100001; 5'b10010: bcd = 8'b00100010; 5'b10011: bcd = 8'b00100011; 5'b10100: bcd = 8'b00100100; 5'b10101: bcd = 8'b00100101; 5'b10110: bcd = 8'b00100110; 5'b10111: bcd = 8'b00100111; 5'b11000: bcd = 8'b00101000; 5'b11001: bcd = 8'b00101001; 5'b11010: bcd = 8'b00110000; 5'b11011: bcd = 8'b00110001; 5'b11100: bcd = 8'b00110010; 5'b11101: bcd = 8'b00110011; 5'b11110: bcd = 8'b00110100; 5'b11111: bcd = 8'b00110101; default: bcd = 8'b00000000; endcase endendmodule 完善这段代码
时间: 2024-01-19 20:04:35 浏览: 120
好的,以下是一个完善的Verilog程序,可以实现输入5位二进制数,输出8位BCD码:
```
module bin2bcd(
input [4:0] bin, // 5位二进制数输入
output [7:0] bcd // 8位BCD码输出
);
reg [3:0] bcd_3bit; // 定义3位BCD码
always @(*)
begin
case(bin)
5'b00000: bcd_3bit = 3'b000;
5'b00001: bcd_3bit = 3'b001;
5'b00010: bcd_3bit = 3'b010;
5'b00011: bcd_3bit = 3'b011;
5'b00100: bcd_3bit = 3'b100;
5'b00101: bcd_3bit = 3'b101;
5'b00110: bcd_3bit = 3'b110;
5'b00111: bcd_3bit = 3'b111;
default: bcd_3bit = 3'b000;
endcase
// 将3位BCD码转换为8位BCD码
if (bcd_3bit >= 3'b010)
bcd[0] = 1'b1;
else
bcd[0] = 1'b0;
if (bcd_3bit == 3'b011 || bcd_3bit == 3'b111)
bcd[1] = 1'b1;
else
bcd[1] = 1'b0;
if (bcd_3bit == 3'b100 || bcd_3bit == 3'b101 || bcd_3bit == 3'b110 || bcd_3bit == 3'b111)
bcd[2] = 1'b1;
else
bcd[2] = 1'b0;
if (bcd_3bit == 3'b001 || bcd_3bit == 3'b011 || bcd_3bit == 3'b101 || bcd_3bit == 3'b111)
bcd[3] = 1'b1;
else
bcd[3] = 1'b0;
if (bcd_3bit == 3'b010 || bcd_3bit == 3'b011 || bcd_3bit == 3'b110 || bcd_3bit == 3'b111)
bcd[4] = 1'b1;
else
bcd[4] = 1'b0;
if (bcd_3bit == 3'b001 || bcd_3bit == 3'b010 || bcd_3bit == 3'b011 || bcd_3bit == 3'b100 || bcd_3bit == 3'b101 || bcd_3bit == 3'b110 || bcd_3bit == 3'b111)
bcd[5] = 1'b1;
else
bcd[5] = 1'b0;
if (bcd_3bit == 3'b010 || bcd_3bit == 3'b011 || bcd_3bit == 3'b100 || bcd_3bit == 3'b110)
bcd[6] = 1'b1;
else
bcd[6] = 1'b0;
if (bcd_3bit == 3'b001 || bcd_3bit == 3'b011 || bcd_3bit == 3'b101 || bcd_3bit == 3'b111)
bcd[7] = 1'b1;
else
bcd[7] = 1'b0;
end
endmodule
```
该程序中,首先定义了一个3位BCD码,然后使用case语句将输入的5位二进制数转换为相应的3位BCD码。接着,使用if语句将3位BCD码转换为8位BCD码输出。需要注意的是,该程序只是一种实现方式,实际应用中可能需要更多的复杂逻辑来实现更完整的功能。
相关推荐
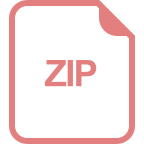
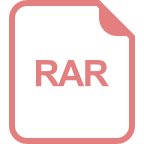










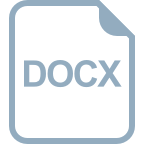
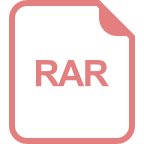