给出一个使用bert模型对中文新闻文本分类的示例,基于tensorflow2.0
时间: 2023-05-22 13:01:27 浏览: 216
以下是一个使用BERT模型对中文新闻文本分类的示例,基于TensorFlow 2.0:
```python
import tensorflow as tf
import tensorflow_hub as hub
import numpy as np
# 加载BERT模型
bert_layer = hub.KerasLayer("https://tfhub.dev/tensorflow/bert_zh_L-12_H-768_A-12/2", trainable=True)
# 构建模型
input_word_ids = tf.keras.layers.Input(shape=(128,), dtype=tf.int32, name="input_word_ids")
input_mask = tf.keras.layers.Input(shape=(128,), dtype=tf.int32, name="input_mask")
segment_ids = tf.keras.layers.Input(shape=(128,), dtype=tf.int32, name="segment_ids")
pooled_output, sequence_output = bert_layer([input_word_ids, input_mask, segment_ids])
output = tf.keras.layers.Dense(10, activation='softmax')(pooled_output)
model = tf.keras.models.Model(inputs=[input_word_ids, input_mask, segment_ids], outputs=output)
# 编译模型
optimizer = tf.keras.optimizers.Adam(learning_rate=2e-5, epsilon=1e-08, clipnorm=1.0)
loss = tf.keras.losses.CategoricalCrossentropy()
metric = tf.keras.metrics.CategoricalAccuracy('accuracy')
model.compile(optimizer=optimizer, loss=loss, metrics=[metric])
# 加载数据集
train_data = tf.data.Dataset.from_tensor_slices((train_input_ids, train_input_masks, train_segment_ids, train_labels)).shuffle(10000).batch(32)
test_data = tf.data.Dataset.from_tensor_slices((test_input_ids, test_input_masks, test_segment_ids, test_labels)).batch(32)
# 训练模型
model.fit(train_data, epochs=5, validation_data=test_data)
# 预测新数据
new_data = ["这是一篇关于科技的新闻", "这是一篇关于体育的新闻"]
new_input_ids = []
new_input_masks = []
new_segment_ids = []
for text in new_data:
tokens = tokenizer.tokenize(text)
tokens = ["[CLS]"] + tokens + ["[SEP]"]
input_ids = tokenizer.convert_tokens_to_ids(tokens)
input_masks = [1] * len(input_ids)
segment_ids = [0] * len(tokens)
padding_length = 128 - len(input_ids)
input_ids = input_ids + ([0] * padding_length)
input_masks = input_masks + ([0] * padding_length)
segment_ids = segment_ids + ([0] * padding_length)
new_input_ids.append(input_ids)
new_input_masks.append(input_masks)
new_segment_ids.append(segment_ids)
new_input_ids = np.array(new_input_ids)
new_input_masks = np.array(new_input_masks)
new_segment_ids = np.array(new_segment_ids)
predictions = model.predict([new_input_ids, new_input_masks, new_segment_ids])
```
这个示例使用了BERT中文预训练模型,对中文新闻文本进行分类。模型的输入是一个长度为128的整数序列,包含了文本的词汇ID、掩码和段ID。模型的输出是一个长度为10的向量,表示文本属于10个不同类别的概率。模型使用交叉熵损失函数和分类精度作为评估指标,使用Adam优化器进行训练。在预测新数据时,需要将新数据转换为模型的输入格式,并使用模型进行预测。
阅读全文
相关推荐
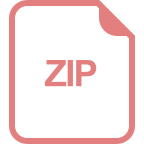

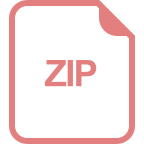
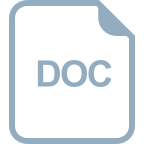
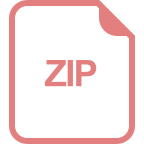
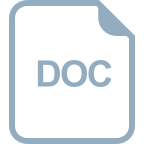
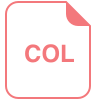
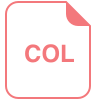
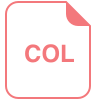
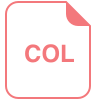
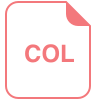
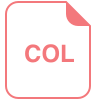
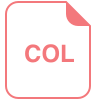
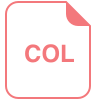
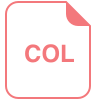
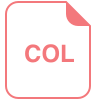
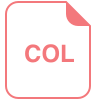