httpclient post 文件下载工具类
时间: 2023-06-20 16:08:38 浏览: 129
以下是一个使用 HttpClient 进行文件下载的工具类示例:
```java
import org.apache.http.HttpEntity;
import org.apache.http.client.HttpClient;
import org.apache.http.client.methods.HttpGet;
import org.apache.http.conn.ClientConnectionManager;
import org.apache.http.impl.client.DefaultHttpClient;
import org.apache.http.params.BasicHttpParams;
import org.apache.http.params.HttpConnectionParams;
import org.apache.http.params.HttpParams;
import org.apache.http.util.EntityUtils;
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
public class FileDownloadUtil {
private static final int TIMEOUT_CONNECTION = 5000; // 连接超时时间
private static final int TIMEOUT_SOCKET = 5000; // 读取超时时间
public static boolean downloadFile(String url, String filePath) {
HttpClient httpClient = null;
HttpGet httpGet = null;
try {
httpClient = getHttpClient();
httpGet = new HttpGet(url);
HttpEntity httpEntity = httpClient.execute(httpGet).getEntity();
if (httpEntity != null) {
FileOutputStream fos = new FileOutputStream(new File(filePath), false);
fos.write(EntityUtils.toByteArray(httpEntity));
fos.flush();
fos.close();
return true;
}
} catch (IOException e) {
e.printStackTrace();
} finally {
if (httpGet != null) {
httpGet.abort();
}
if (httpClient != null) {
ClientConnectionManager mgr = httpClient.getConnectionManager();
if (mgr != null) {
mgr.shutdown();
}
}
}
return false;
}
private static HttpClient getHttpClient() {
HttpParams params = new BasicHttpParams();
HttpConnectionParams.setConnectionTimeout(params, TIMEOUT_CONNECTION);
HttpConnectionParams.setSoTimeout(params, TIMEOUT_SOCKET);
return new DefaultHttpClient(params);
}
}
```
使用示例:
```java
String url = "http://example.com/example.pdf";
String filePath = "/path/to/file/example.pdf";
boolean success = FileDownloadUtil.downloadFile(url, filePath);
if (success) {
System.out.println("文件下载成功!");
} else {
System.out.println("文件下载失败!");
}
```
需要注意的是,在使用 HttpClient 进行网络请求时,需要在 AndroidManifest.xml 文件中添加网络访问权限:
```xml
<uses-permission android:name="android.permission.INTERNET" />
```
阅读全文
相关推荐
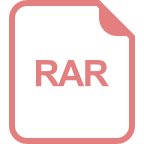
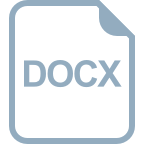
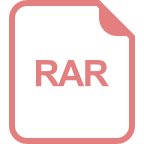
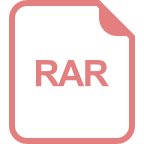
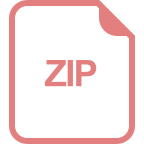



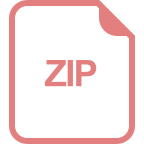
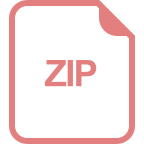
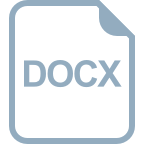
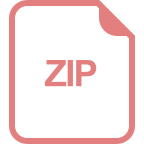
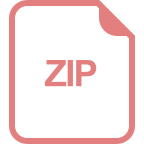
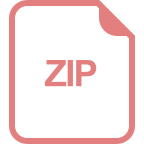
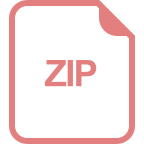
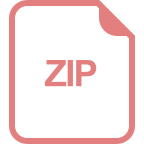