label_data = (label_data == 1).astype(np.uint8)代码解释
时间: 2024-04-01 18:31:39 浏览: 18
这行代码的作用是将`label_data`中的所有元素值为1的元素转换为整数类型np.uint8的1,其余元素转换为整数类型np.uint8的0。具体来说,该行代码使用了numpy中的astype函数,将`label_data`先转换为布尔型数组,值为1的元素为True,值为0的元素为False,然后将True转换为np.uint8类型的1,False转换为np.uint8类型的0。这行代码常用于将二分类标签转换为0和1。
相关问题
解释每一句代码for file in os.listdir(os.path.join(data_path, 'Training Batch 2')): if file.endswith('.nii'): # 读取nii文件 label_nii = nib.load(os.path.join(data_path, 'Training Batch 2', file)) label_array = label_nii.get_fdata() # 将3D标签转换为2D标签 for i in range(label_array.shape[2]): label_slice = label_array[:, :, i]* 122 label_slice[label_slice == 1] = 255 label_slice[label_slice == 2] = 128 # 保存标签 save_name = file.split('.')[0] + '_' + str(i) + '.png' save_path_label = os.path.join(save_path, 'label', save_name) Image.fromarray(label_slice.astype(np.uint8)).save(save_path_label)
好的,我来解释一下这段代码的作用:
```
for file in os.listdir(os.path.join(data_path, 'Training Batch 2')):
```
这一行代码是遍历指定文件夹下的所有文件。具体来说,它使用`os.listdir`函数列出了指定路径下的所有文件名,然后使用`os.path.join`函数将路径和文件名组合起来,得到每个文件的完整路径,并将其存储在变量`file`中。
```
if file.endswith('.nii'):
```
这一行代码是判断当前文件是否是以`.nii`结尾的NIfTI格式文件。如果是,则继续执行下面的代码;否则跳过当前循环,继续遍历下一个文件。
```
label_nii = nib.load(os.path.join(data_path, 'Training Batch 2', file))
```
这一行代码使用`nib.load`函数读取NIfTI格式的文件,并将其存储在变量`label_nii`中。
```
label_array = label_nii.get_fdata()
```
这一行代码是将`label_nii`中的数据转换为numpy数组,并将其存储在变量`label_array`中。
```
for i in range(label_array.shape[2]):
```
这一行代码是遍历`label_array`的第三个维度,即z轴方向。这里使用`label_array.shape[2]`获取了数组在z轴方向上的长度,然后使用`range`函数生成了一个从0到该长度减1的整数序列,依次赋值给变量`i`。
```
label_slice = label_array[:, :, i]* 122
label_slice[label_slice == 1] = 255
label_slice[label_slice == 2] = 128
```
这三行代码是将`label_array`中第i个切片提取出来,并对其进行一些处理。具体来说,它们将该切片中所有值为1的像素点设为255,将所有值为2的像素点设为128,并将该切片中所有像素点的值乘以122,最后将处理后的结果存储在变量`label_slice`中。
```
save_name = file.split('.')[0] + '_' + str(i) + '.png'
save_path_label = os.path.join(save_path, 'label', save_name)
Image.fromarray(label_slice.astype(np.uint8)).save(save_path_label)
```
这三行代码是将处理后的标签保存为PNG格式的文件。具体来说,它们使用`file.split`函数将文件名按照`.`分割,取第一部分作为文件名的前缀,然后再加上下划线和当前切片的索引,组成新的文件名。然后使用`os.path.join`函数将文件保存路径和文件名组合起来,得到完整的保存路径,并将其存储在变量`save_path_label`中。最后,使用`Image.fromarray`函数将`label_slice`转换为PIL图像对象,并将其保存到`save_path_label`路径下。
代码import os import numpy as np import nibabel as nib from PIL import Image # 创建保存路径 save_path = 'C:/Users/Administrator/Desktop/2D-LiTS2017' if not os.path.exists(save_path): os.makedirs(save_path) if not os.path.exists(os.path.join(save_path, 'image')): os.makedirs(os.path.join(save_path, 'image')) if not os.path.exists(os.path.join(save_path, 'label')): os.makedirs(os.path.join(save_path, 'label')) # 加载数据集 data_path = 'D:/BaiduNetdiskDownload/LiTS2017' img_path = os.path.join(data_path, 'Training Batch 1') label_path = os.path.join(data_path, 'Training Batch 2') # 转换图像 for file in sorted(os.listdir(img_path)): if file.endswith('.nii'): img_file = os.path.join(img_path, file) img = nib.load(img_file).get_fdata() img = np.transpose(img, (2, 0, 1)) # 转换为z, x, y for i in range(img.shape[0]): img_slice = img[i, :, :] img_slice = (img_slice - np.min(img_slice)) / (np.max(img_slice) - np.min(img_slice)) * 255 # 归一化到0-255 img_slice = img_slice.astype(np.uint8) img_slice = np.stack([img_slice]*3, axis=2) # 转换为三通道图像 img_name = file[:-4] + '' + str(i).zfill(3) + '.png' img_file_save = os.path.join(save_path, 'image', img_name) Image.fromarray(img_slice).save(img_file_save) # 转换标签 for file in sorted(os.listdir(label_path)): if file.endswith('.nii'): label_file = os.path.join(label_path, file) label = nib.load(label_file).get_fdata() label = np.transpose(label, (2, 0, 1)) # 转换为z, x, y for i in range(label.shape[0]): label_slice = label[i, :, :] label_slice[label_slice == 1] = 255 # 肝脏灰度值设为255 label_slice[label_slice == 2] = 128 # 肝脏肿瘤灰度值设为128 label_slice = label_slice.astype(np.uint8) label_name = file[:-4] + '' + str(i).zfill(3) + '.png' label_file_save = os.path.join(save_path, 'label', label_name) Image.fromarray(label_slice).save(label_file_save)出现scaled = scaled.astype(np.promote_types(scaled.dtype, dtype), copy=False) MemoryError错误,怎么修改?给出完整代码
这个错误通常意味着你的计算机没有足够的内存来处理数组。可能需要减少转换图像的尺寸或者使用更高效的算法。以下是一个可能的修改建议:
```python
import os
import numpy as np
import nibabel as nib
from PIL import Image
# 创建保存路径
save_path = 'C:/Users/Administrator/Desktop/2D-LiTS2017'
if not os.path.exists(save_path):
os.makedirs(save_path)
if not os.path.exists(os.path.join(save_path, 'image')):
os.makedirs(os.path.join(save_path, 'image'))
if not os.path.exists(os.path.join(save_path, 'label')):
os.makedirs(os.path.join(save_path, 'label'))
# 加载数据集
data_path = 'D:/BaiduNetdiskDownload/LiTS2017'
img_path = os.path.join(data_path, 'Training Batch 1')
label_path = os.path.join(data_path, 'Training Batch 2')
# 转换图像
for file in sorted(os.listdir(img_path)):
if file.endswith('.nii'):
img_file = os.path.join(img_path, file)
img = nib.load(img_file).get_fdata()
img = np.transpose(img, (2, 0, 1)) # 转换为z, x, y
for i in range(img.shape[0]):
img_slice = img[i, :, :]
img_slice = (img_slice - np.min(img_slice)) / (np.max(img_slice) - np.min(img_slice)) * 255 # 归一化到0-255
img_slice = img_slice.astype(np.uint8)
img_slice = np.stack([img_slice]*3, axis=2) # 转换为三通道图像
#减少图像大小
img_slice = np.array(Image.fromarray(img_slice).resize((512, 512), resample=Image.BICUBIC))
img_name = file[:-4] + '' + str(i).zfill(3) + '.png'
img_file_save = os.path.join(save_path, 'image', img_name)
Image.fromarray(img_slice).save(img_file_save)
# 转换标签
for file in sorted(os.listdir(label_path)):
if file.endswith('.nii'):
label_file = os.path.join(label_path, file)
label = nib.load(label_file).get_fdata()
label = np.transpose(label, (2, 0, 1)) # 转换为z, x, y
for i in range(label.shape[0]):
label_slice = label[i, :, :]
label_slice[label_slice == 1] = 255 # 肝脏灰度值设为255
label_slice[label_slice == 2] = 128 # 肝脏肿瘤灰度值设为128
label_slice = label_slice.astype(np.uint8)
label_name = file[:-4] + '' + str(i).zfill(3) + '.png'
label_file_save = os.path.join(save_path, 'label', label_name)
Image.fromarray(label_slice).save(label_file_save)
```
这里我们使用了 `PIL.Image` 中的 `resize()` 函数来减少图像大小。`resample=Image.BICUBIC` 用于指定插值方法,这里使用了 Bicubic 插值。这个方法可以保留图像的细节,并且可以减少内存使用。你也可以尝试使用其他插值方法。
相关推荐









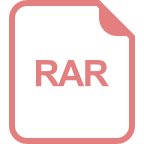
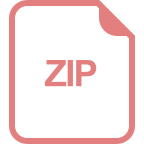