python any()
时间: 2024-05-02 16:21:45 浏览: 81
The `any()` function in Python takes an iterable (list, tuple, dictionary, etc.) as argument and returns `True` if at least one element in the iterable is `True`, otherwise it returns `False`.
Syntax:
```python
any(iterable)
```
Example:
```python
# using any() with a list
lst = [False, True, False, True]
print(any(lst)) # True
# using any() with a tuple
tpl = (0, '', False)
print(any(tpl)) # False
# using any() with a dictionary
dct = {'a': 1, 'b': 0, 'c': 2}
print(any(dct)) # True (since 'a': 1 is True)
# using any() with a string
string = 'hello'
print(any(string)) # True
```
Note: If the iterable is empty, `any()` will return `False`.
相关问题
python any
`any` 是一个 Python 内置函数,用于判断可迭代对象中是否有任何一个元素为真(非零、非空、非 None)。它接受一个可迭代对象作为参数,并返回一个布尔值。如果可迭代对象中至少有一个元素为真,则返回 `True`,否则返回 `False`。
下面是一个示例:
```python
numbers = [0, 1, 2, 3, 4]
result = any(numbers)
print(result) # 输出 True,因为列表中至少有一个元素(1)为真
```
在这个示例中,`any` 函数检查列表 `numbers` 中的元素是否存在至少一个为真的情况。由于列表中的元素包括零和其他非零值,所以 `any` 函数返回 `True`。
请注意,如果可迭代对象为空,则 `any` 函数也会返回 `False`。例如:
```python
empty_list = []
result = any(empty_list)
print(result) # 输出 False,因为列表为空
```
这是 `any` 函数的基本用法。如果有其他问题,请随时提问!
python any用法
`any()` 是 Python 内置函数之一,用于判断一个可迭代对象中是否存在任意一个元素满足指定条件。它的语法如下:
```python
any(iterable)
```
其中,`iterable` 是一个可迭代对象,例如列表、元组、集合等。`any()` 函数将会遍历 `iterable` 中的所有元素,并返回一个布尔值,表示是否存在任意一个元素满足指定条件。
以下是 `any()` 函数的一些示例用法:
```python
# 示例1:检查列表中是否存在奇数
nums = [2, 4, 6, 8, 9, 10]
has_odd = any(num % 2 != 0 for num in nums)
print(has_odd) # True
# 示例2:检查字符串中是否存在小写字母
s = "Hello, World!"
has_lowercase = any(c.islower() for c in s)
print(has_lowercase) # True
# 示例3:检查集合中是否存在负数
nums_set = {1, 2, 3, -4, 5}
has_negative = any(num < 0 for num in nums_set)
print(has_negative) # True
```
在示例1中,我们使用了一个生成器表达式来检查列表中是否存在奇数。在示例2中,我们使用了字符串的 `islower()` 方法来检查字符串中是否存在小写字母。在示例3中,我们使用了集合和比较运算符来检查集合中是否存在负数。
阅读全文
相关推荐
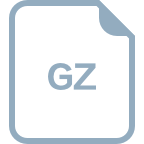
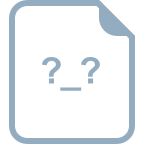
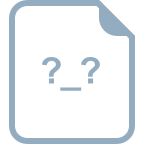













