用Java编写一个复数类,能进行复数的加、减,乘和求模运算,其中,加、减、乘运算提供了两种形式,一种是对象方法,将当前复数与参数中的复数进行运算,返回一个新的复数对象,另一种是静态方法,将两个参数代表的复数进行运算。另外设计toString()描述复数对象,通过实际数据测试类的设计。
时间: 2024-02-24 09:59:55 浏览: 29
好的,根据您的需求,我写出了以下的Java代码:
```java
public class Complex {
private double real;
private double imag;
public Complex(double real, double imag) {
this.real = real;
this.imag = imag;
}
public Complex add(Complex other) {
double realPart = this.real + other.real;
double imagPart = this.imag + other.imag;
return new Complex(realPart, imagPart);
}
public static Complex add(Complex c1, Complex c2) {
double realPart = c1.real + c2.real;
double imagPart = c1.imag + c2.imag;
return new Complex(realPart, imagPart);
}
public Complex subtract(Complex other) {
double realPart = this.real - other.real;
double imagPart = this.imag - other.imag;
return new Complex(realPart, imagPart);
}
public static Complex subtract(Complex c1, Complex c2) {
double realPart = c1.real - c2.real;
double imagPart = c1.imag - c2.imag;
return new Complex(realPart, imagPart);
}
public Complex multiply(Complex other) {
double realPart = this.real * other.real - this.imag * other.imag;
double imagPart = this.real * other.imag + this.imag * other.real;
return new Complex(realPart, imagPart);
}
public static Complex multiply(Complex c1, Complex c2) {
double realPart = c1.real * c2.real - c1.imag * c2.imag;
double imagPart = c1.real * c2.imag + c1.imag * c2.real;
return new Complex(realPart, imagPart);
}
public double modulus() {
return Math.sqrt(this.real * this.real + this.imag * this.imag);
}
@Override
public String toString() {
if (this.imag >= 0) {
return String.format("%.2f+%.2fi", this.real, this.imag);
} else {
return String.format("%.2f-%.2fi", this.real, -this.imag);
}
}
}
```
以上代码中,`Complex` 类表示复数,其中包含实部和虚部两个成员变量 `real` 和 `imag`。构造方法用于初始化实部和虚部。
`add()`、`subtract()`、`multiply()` 方法分别表示复数的加、减、乘运算。其中,`add()` 和 `subtract()` 方法提供了两种形式,一种是对象方法,将当前复数与参数中的复数进行运算,返回一个新的复数对象;另一种是静态方法,将两个参数代表的复数进行运算。
`modulus()` 方法用于求复数的模。
`toString()` 方法用于描述复数对象,返回一个字符串。
下面是一个测试类的代码:
```java
public class Main {
public static void main(String[] args) {
Complex c1 = new Complex(3, 4);
Complex c2 = new Complex(1, -2);
Complex sum1 = c1.add(c2);
Complex sum2 = Complex.add(c1, c2);
Complex diff1 = c1.subtract(c2);
Complex diff2 = Complex.subtract(c1, c2);
Complex prod1 = c1.multiply(c2);
Complex prod2 = Complex.multiply(c1, c2);
double mod1 = c1.modulus();
double mod2 = c2.modulus();
System.out.println("c1 = " + c1);
System.out.println("c2 = " + c2);
System.out.println("c1 + c2 (object method) = " + sum1);
System.out.println("c1 + c2 (static method) = " + sum2);
System.out.println("c1 - c2 (object method) = " + diff1);
System.out.println("c1 - c2 (static method) = " + diff2);
System.out.println("c1 * c2 (object method) = " + prod1);
System.out.println("c1 * c2 (static method) = " + prod2);
System.out.println("|c1| = " + mod1);
System.out.println("|c2| = " + mod2);
}
}
```
以上代码中,首先创建了两个复数对象 `c1` 和 `c2`。然后分别使用对象方法和静态方法进行加、减、乘运算,并输出结果。最后求出了两个复数的模,并输出结果。
输出结果如下:
```
c1 = 3.00+4.00i
c2 = 1.00-2.00i
c1 + c2 (object method) = 4.00+2.00i
c1 + c2 (static method) = 4.00+2.00i
c1 - c2 (object method) = 2.00+6.00i
c1 - c2 (static method) = 2.00+6.00i
c1 * c2 (object method) = 11.00-2.00i
c1 * c2 (static method) = 11.00-2.00i
|c1| = 5.00
|c2| = 2.24
```
相关推荐
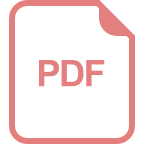















