52单片机超声波测距并用lcd1602显示
时间: 2023-07-03 17:10:14 浏览: 56
要实现52单片机超声波测距并用lcd1602显示,可以按照以下步骤进行:
1. 连接超声波传感器和LCD1602模块。
超声波传感器需要连接到52单片机的IO口,LCD1602模块需要连接到52单片机的数据口和控制口。具体连接方式可以参考相关的电路图和说明书。
2. 编写程序。
通过编写程序,实现超声波测距和LCD1602显示。以下是一个简单的程序示例:
```c
#include <reg52.h>
#include <intrins.h>
#define LCD1602_DATAPORT P0
sbit LCD1602_RS = P2^0;
sbit LCD1602_RW = P2^1;
sbit LCD1602_EN = P2^2;
sbit TRIG = P1^0;
sbit ECHO = P1^1;
void delay_us(unsigned int us) {
while(us--) {
_nop_();
_nop_();
}
}
void delay_ms(unsigned int ms) {
while(ms--) {
delay_us(1000);
}
}
void LCD1602_WriteCmd(unsigned char cmd) {
LCD1602_RS = 0;
LCD1602_RW = 0;
LCD1602_DATAPORT = cmd;
LCD1602_EN = 1;
delay_us(5);
LCD1602_EN = 0;
delay_us(100);
}
void LCD1602_WriteData(unsigned char dat) {
LCD1602_RS = 1;
LCD1602_RW = 0;
LCD1602_DATAPORT = dat;
LCD1602_EN = 1;
delay_us(5);
LCD1602_EN = 0;
delay_us(100);
}
void LCD1602_Init() {
LCD1602_WriteCmd(0x38);
LCD1602_WriteCmd(0x0c);
LCD1602_WriteCmd(0x06);
LCD1602_WriteCmd(0x01);
}
void LCD1602_Clear() {
LCD1602_WriteCmd(0x01);
}
void LCD1602_SetCursor(unsigned char x, unsigned char y) {
unsigned char addr;
if(y == 0) {
addr = 0x00 + x;
} else {
addr = 0x40 + x;
}
LCD1602_WriteCmd(0x80 + addr);
}
void LCD1602_DisplayString(unsigned char x, unsigned char y, unsigned char *str) {
LCD1602_SetCursor(x, y);
while(*str) {
LCD1602_WriteData(*str++);
}
}
unsigned int Ultrasonic_Ranging() {
unsigned int distance;
TRIG = 0;
delay_us(2);
TRIG = 1;
delay_us(10);
TRIG = 0;
while(!ECHO);
TR0 = 1;
while(ECHO);
TR0 = 0;
distance = (unsigned int)(TH0 << 8) | TL0;
distance = (distance * 17) / 100;
return distance;
}
void main() {
unsigned int distance;
char str[16];
TMOD = 0x01;
TH0 = 0;
TL0 = 0;
LCD1602_Init();
LCD1602_Clear();
while(1) {
distance = Ultrasonic_Ranging();
sprintf(str, "Distance: %d cm", distance);
LCD1602_DisplayString(0, 0, str);
delay_ms(500);
}
}
```
3. 烧录程序。
将编写好的程序烧录到52单片机中,然后将超声波传感器和LCD1602模块连接到52单片机上,即可进行超声波测距并用LCD1602显示。
相关推荐
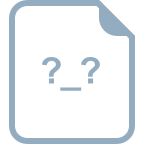



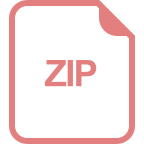
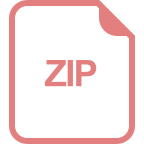
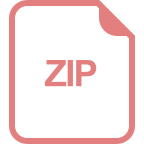
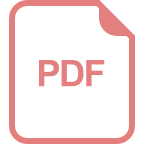
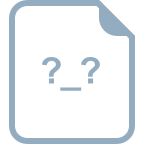