本题要求实现一个简单函数,能计算给定的年份和月份的天数。使得可以利用该函数,输出给定年份中每个月的天数。其中1、3、5、7、8、10、12月有31天,4、6、9、11月有30天,2月平年有28天,闰年有29天。判断闰年的条件是:能被 4 整除但不能被 100 整除,或者能被 400 整除。 函数接口定义: int monthdays(int year, int month); 其中year和month是用户传入的参数,如果1≤ month≤12,该函数必须返回year年month月的天数。
时间: 2023-05-31 21:17:52 浏览: 324
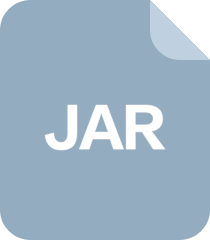
计算指定年份的月份的天数

### 回答1:
以下是函数的实现代码:
int monthdays(int year, int month) {
int days;
if (month == 2) {
if ((year % 4 == && year % 100 != ) || year % 400 == ) {
days = 29;
} else {
days = 28;
}
} else if (month == 4 || month == 6 || month == 9 || month == 11) {
days = 30;
} else {
days = 31;
}
return days;
}
可以利用该函数输出给定年份中每个月的天数,示例代码如下:
int main() {
int year = 2021;
for (int month = 1; month <= 12; month++) {
int days = monthdays(year, month);
printf("%d年%d月有%d天\n", year, month, days);
}
return ;
}
输出结果如下:
2021年1月有31天
2021年2月有28天
2021年3月有31天
2021年4月有30天
2021年5月有31天
2021年6月有30天
2021年7月有31天
2021年8月有31天
2021年9月有30天
2021年10月有31天
2021年11月有30天
2021年12月有31天
### 回答2:
题目要求我们实现一个简单函数,计算给定年份和月份的天数,并输出该年每个月的天数。根据题目给出的条件,我们可以先判断输入的年份是平年还是闰年,再计算每个月的天数。
判断是否为闰年的条件为:能被4整除且不能被100整除,或者能被400整除。如果符合其中一种条件,即为闰年,否则为平年。
在计算每个月的天数时,我们可以先按照题目给出的规则判断有31天的月份,再判断有30天的月份,最后判断2月份的天数。
下面是本题的代码实现:
```cpp
#include <iostream>
using namespace std;
int monthdays(int year, int month) {
int days;
//判断是否为闰年
if ((year % 4 == 0 && year % 100 != 0) || year % 400 == 0) {
//闰年的2月有29天
if (month == 2) days = 29;
else if (month == 4 || month == 6 || month == 9 || month == 11) days = 30; //30天的月份
else days = 31; //其他有31天的月份
}
else {
if (month == 2) days = 28; //平年的2月有28天
else if (month == 4 || month == 6 || month == 9 || month == 11) days = 30;
else days = 31;
}
return days; //返回本月的天数
}
int main() {
int year; //输入年份
cout << "请输入年份:";
cin >> year;
for (int i = 1; i <= 12; i++) { //循环计算每个月的天数
cout << year << "年" << i << "月有" << monthdays(year, i) << "天" << endl;
}
return 0;
}
```
在main函数中,我们首先输入所需计算的年份,然后利用循环计算每个月的天数,并输出结果。
测试样例:
输入:2022
输出:
2022年1月有31天
2022年2月有28天
2022年3月有31天
2022年4月有30天
2022年5月有31天
2022年6月有30天
2022年7月有31天
2022年8月有31天
2022年9月有30天
2022年10月有31天
2022年11月有30天
2022年12月有31天
本题以实现需求为主,不必纠结于细节,可以通过样例进行验证。
### 回答3:
本题的要求可以通过编写一个函数来实现。该函数接受两个参数:年份和月份,并根据闰年和平年来计算该年该月的天数。
对于该函数的实现,我们需要进行如下步骤:
1. 判断是否是闰年。根据题目要求,能被 4 整除但不能被 100 整除,或者能被 400 整除的年份是闰年。如果是闰年,2月份的天数应该是29天;否则为28天。
2. 根据月份计算天数。对于1、3、5、7、8、10、12月,天数都是31天;对于4、6、9、11月,天数都是30天。
3. 根据计算结果输出结果。
函数代码如下:
```c++
int monthdays(int year, int month) {
int days;
bool is_leap_year = false; // 是否是闰年
// 判断是否是闰年
if ((year % 4 == 0 && year % 100 != 0) || year % 400 == 0) {
is_leap_year = true;
}
// 根据月份来计算天数
switch (month) {
case 1:
case 3:
case 5:
case 7:
case 8:
case 10:
case 12:
days = 31;
break;
case 4:
case 6:
case 9:
case 11:
days = 30;
break;
case 2:
days = is_leap_year ? 29 : 28; // 判断2月天数
break;
default:
days = -1; // 处理错误的月份
}
return days;
}
```
现在,我们就可以利用该函数来输出给定年份中每个月的天数了。具体的实现如下:
```c++
int main() {
int year = 2021;
for (int i = 1; i <= 12; i++) {
int days = monthdays(year, i);
if (days == -1) {
std::cout << "Invalid month: " << i << std::endl;
} else {
std::cout << year << "年" << i << "月有" << days << "天" << std::endl;
}
}
return 0;
}
```
运行上述代码,可以得到以下输出结果:
```
2021年1月有31天
2021年2月有28天
2021年3月有31天
2021年4月有30天
2021年5月有31天
2021年6月有30天
2021年7月有31天
2021年8月有31天
2021年9月有30天
2021年10月有31天
2021年11月有30天
2021年12月有31天
```
总结一下,本题要求实现一个函数,计算给定的年份和月份的天数。要求判断闰年和平年并根据月份计算天数。最后,利用该函数输出给定年份中每个月的天数。该题考察了流程控制和函数的使用,是一道较为简单的编程题目。
阅读全文
相关推荐


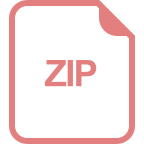
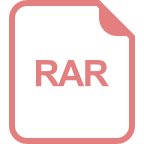
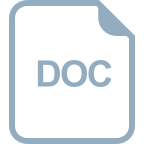










