Android Studio记事本代码
时间: 2023-07-14 20:12:30 浏览: 114
以下是一个基本的 Android Studio 记事本应用的代码示例:
MainActivity.java:
```java
package com.example.notepad;
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.EditText;
import android.widget.Toast;
import java.io.BufferedReader;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStreamReader;
public class MainActivity extends AppCompatActivity {
EditText editText;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
editText = findViewById(R.id.editText);
}
public void saveFile(View view) {
String text = editText.getText().toString();
FileOutputStream fileOutputStream = null;
try {
fileOutputStream = openFileOutput("note.txt", MODE_PRIVATE);
fileOutputStream.write(text.getBytes());
Toast.makeText(this, "Note saved successfully!", Toast.LENGTH_SHORT).show();
} catch (Exception e) {
e.printStackTrace();
Toast.makeText(this, "Error saving note!", Toast.LENGTH_SHORT).show();
} finally {
try {
fileOutputStream.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
public void loadFile(View view) {
FileInputStream fileInputStream = null;
try {
fileInputStream = openFileInput("note.txt");
InputStreamReader inputStreamReader = new InputStreamReader(fileInputStream);
BufferedReader bufferedReader = new BufferedReader(inputStreamReader);
StringBuilder stringBuilder = new StringBuilder();
String text;
while ((text = bufferedReader.readLine()) != null) {
stringBuilder.append(text).append("\n");
}
editText.setText(stringBuilder.toString());
Toast.makeText(this, "Note loaded successfully!", Toast.LENGTH_SHORT).show();
} catch (Exception e) {
e.printStackTrace();
Toast.makeText(this, "Error loading note!", Toast.LENGTH_SHORT).show();
} finally {
try {
fileInputStream.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
public void deleteFile(View view) {
File file = new File(getFilesDir(), "note.txt");
if (file.exists()) {
if (file.delete()) {
editText.setText("");
Toast.makeText(this, "Note deleted successfully!", Toast.LENGTH_SHORT).show();
} else {
Toast.makeText(this, "Error deleting note!", Toast.LENGTH_SHORT).show();
}
} else {
Toast.makeText(this, "Note does not exist!", Toast.LENGTH_SHORT).show();
}
}
}
```
activity_main.xml:
```xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<EditText
android:id="@+id/editText"
android:layout_width="match_parent"
android:layout_height="0dp"
android:layout_weight="1"
android:gravity="top|start"
android:hint="Write your note here"
android:padding="16dp"
android:textSize="18sp" />
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal">
<Button
android:id="@+id/buttonSave"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="Save"
android:onClick="saveFile" />
<Button
android:id="@+id/buttonLoad"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="Load"
android:onClick="loadFile" />
<Button
android:id="@+id/buttonDelete"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="Delete"
android:onClick="deleteFile" />
</LinearLayout>
</LinearLayout>
```
此示例实现了在 Android Studio 中创建一个简单的记事本应用,其中有三个按钮:保存、加载和删除。用户可以在文本框中输入文本,然后单击“保存”按钮将该文本保存到文件中,单击“加载”按钮将该文本从文件中读取并在文本框中显示,单击“删除”按钮将删除该文件。
阅读全文
相关推荐
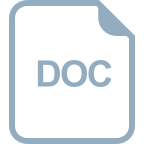
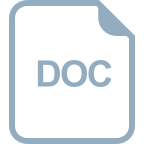
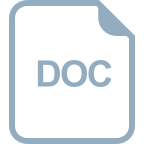















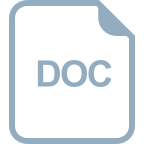