设计代码完成任务:假设银行有K个窗口提供服务,窗口前设一条黄线,所有顾客按到达时间在黄线后排成一条长龙。当有窗口空闲时,下一位顾客即去该窗口处理事务。当有多个窗口可选择时,假设顾客总是选择编号最小的窗口。 有些银行会给VIP客户以各种优惠服务,例如专门开辟VIP窗口。为了最大限度地利用资源,VIP窗口的服务机制定义为:当队列中没有VIP客户时,该窗口为普通顾客服务;当该窗口空闲并且队列中有VIP客户在等待时,排在最前面的VIP客户享受该窗口的服务。同时,当轮到某VIP客户出列时,若VIP窗口非空,该客户可以选择空闲的普通窗口;否则一定选择VIP窗口。
时间: 2023-05-29 15:03:31 浏览: 146
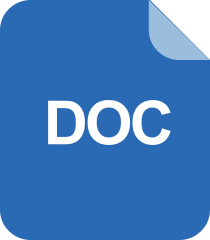
基于队列的银行窗口设置模拟
假设银行有K个窗口提供服务,可以使用队列实现顾客排队等待服务的功能。为了实现VIP客户的优惠服务,可以使用两个队列,一个队列存放普通顾客,一个队列存放VIP客户。同时,需要记录每个窗口的状态,包括是否空闲和正在服务的顾客类型(普通/ VIP)。
具体实现如下:
首先定义一个顾客类,包括顾客编号、到达时间和是否为VIP客户等属性。
```python
class Customer:
def __init__(self, id, arrive_time, is_VIP):
self.id = id
self.arrive_time = arrive_time
self.is_VIP = is_VIP
```
然后定义一个银行类,包括窗口数、普通队列、VIP队列和窗口状态等属性。其中,窗口状态用一个列表来表示,每个元素包括窗口是否空闲和正在服务的顾客编号和类型。
```python
class Bank:
def __init__(self, K):
self.K = K
self.normal_queue = []
self.VIP_queue = []
self.windows = [[True, None, None] for i in range(K)]
```
接着实现顾客到达和离开的功能。当顾客到达时,根据是否为VIP客户选择加入普通队列还是VIP队列。当顾客离开时,需要将其从窗口状态和队列中移除,并将下一个顾客送到该窗口。
```python
class Bank:
...
def customer_arrive(self, id, arrive_time, is_VIP):
c = Customer(id, arrive_time, is_VIP)
if is_VIP:
self.VIP_queue.append(c)
else:
self.normal_queue.append(c)
self.serve_customer()
def customer_leave(self, time):
for w in self.windows:
if w[1] is not None and w[1].arrive_time + 60 <= time:
# 顾客服务时间结束,窗口空闲
w[0] = True
w[1] = None
# 将下一个顾客送到该窗口
self.serve_customer()
self.serve_customer()
def serve_customer(self):
# 遍历窗口,找到空闲窗口
for i in range(self.K):
if self.windows[i][0]:
# VIP队列中有VIP顾客,优先服务
if self.VIP_queue and self.VIP_queue[0].arrive_time <= time:
self.windows[i][0] = False
self.windows[i][1] = self.VIP_queue.pop(0)
# VIP窗口空闲并且有VIP顾客在等待,优先服务
elif i == 0 and self.normal_queue and not self.VIP_queue:
self.windows[i][0] = False
self.windows[i][1] = self.normal_queue.pop(0)
# 普通队列中有顾客,服务
elif self.normal_queue:
self.windows[i][0] = False
self.windows[i][1] = self.normal_queue.pop(0)
```
最后,完成整个银行服务过程的模拟。假设有N个顾客到达,可以按照到达时间的先后顺序依次调用customer_arrive()函数。同时,需要设置一个计时器,每秒钟调用一次customer_leave()函数,模拟顾客离开和下一个顾客到达的过程。
```python
class Bank:
...
def simulate(self, customers):
for c in customers:
self.customer_arrive(*c)
time = 0
while True:
if not self.normal_queue and not self.VIP_queue:
break
self.customer_leave(time)
time += 1
```
完整代码如下:
阅读全文
相关推荐
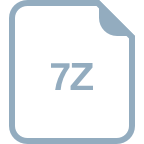
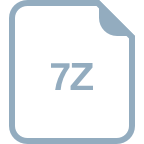












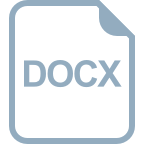
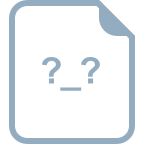
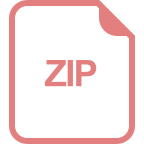
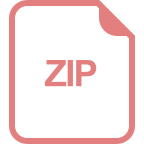
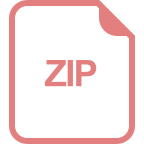