linux系统修改文件时显示Please check that you typed the location correctly and try again.怎么解决
时间: 2024-04-20 07:23:29 浏览: 7
这个问题通常会出现在以下几种情况下:
1. 路径错误:请确保你输入的文件路径是正确的。你可以使用 `ls` 命令来检查文件所在的目录是否存在,然后再次确认路径。
2. 权限问题:如果你没有足够的权限来修改文件,你会看到这个错误。你可以使用 `ls -l` 命令来查看文件的权限和所有者,然后使用 `chmod` 命令来更改文件的权限。
3. 文件不存在:如果文件实际上不存在,你也会看到这个错误。你可以使用 `ls` 命令来检查文件是否存在。
如果以上方法都没有解决问题,你可以尝试以超级用户身份尝试修改文件,即使用 `sudo` 命令。例如:`sudo vim /path/to/file`。请确保在使用 `sudo` 命令时谨慎操作,以免对系统造成损害。
相关问题
write java。Once you have checked that your methods readLine and readPosInt work correctly, remove all the code inside the main method of the CLI class so that the main method is empty again. In the rest of this question, use the readLine and readPosInt methods every time your program needs to read a string or an integer from the user. In the empty main method of the CLI class, create a single Library object with the name "hhh Library". The main method of the CLI class must then print a menu that allows the user of your system to do six different actions that involve the library object, and your program must then read an integer from the user that indicates which action must be performed by the program (see below for the details about each action). Use the readPosInt method to print the menu (give the string for the menu as the argument of readPosInt) and to read the integer typed by the user.
Here is an example implementation of the CLI class with the required functionality:
```java
import java.util.Scanner;
public class CLI {
private static final String MENU = "1. Add a book\n2. Remove a book\n3. Search for a book\n4. Borrow a book\n5. Return a book\n6. Quit";
private static Library library;
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
library = new Library("hhh Library");
int choice = -1;
while (choice != 6) {
System.out.println(MENU);
choice = readPosInt(scanner, "Enter your choice: ");
switch (choice) {
case 1:
addBook(scanner);
break;
case 2:
removeBook(scanner);
break;
case 3:
searchBook(scanner);
break;
case 4:
borrowBook(scanner);
break;
case 5:
returnBook(scanner);
break;
case 6:
System.out.println("Goodbye!");
break;
default:
System.out.println("Invalid choice. Please try again.");
break;
}
}
}
private static void addBook(Scanner scanner) {
String title = readLine(scanner, "Enter the title of the book: ");
String author = readLine(scanner, "Enter the author of the book: ");
int year = readPosInt(scanner, "Enter the publication year of the book: ");
library.addBook(new Book(title, author, year));
System.out.println("Book added successfully!");
}
private static void removeBook(Scanner scanner) {
String title = readLine(scanner, "Enter the title of the book to remove: ");
boolean removed = library.removeBook(title);
if (removed) {
System.out.println("Book removed successfully!");
} else {
System.out.println("Book not found.");
}
}
private static void searchBook(Scanner scanner) {
String searchTerm = readLine(scanner, "Enter a search term for the title or author of the book: ");
Book[] results = library.searchBooks(searchTerm);
if (results.length == 0) {
System.out.println("No results found.");
} else {
System.out.println("Search results:");
for (Book book : results) {
System.out.println(book);
}
}
}
private static void borrowBook(Scanner scanner) {
String title = readLine(scanner, "Enter the title of the book to borrow: ");
boolean borrowed = library.borrowBook(title);
if (borrowed) {
System.out.println("Book borrowed successfully!");
} else {
System.out.println("Book not available for borrowing.");
}
}
private static void returnBook(Scanner scanner) {
String title = readLine(scanner, "Enter the title of the book to return: ");
boolean returned = library.returnBook(title);
if (returned) {
System.out.println("Book returned successfully!");
} else {
System.out.println("Book not found or not borrowed.");
}
}
private static String readLine(Scanner scanner, String message) {
System.out.print(message);
return scanner.nextLine();
}
private static int readPosInt(Scanner scanner, String message) {
int num = -1;
while (num < 0) {
System.out.print(message);
try {
num = Integer.parseInt(scanner.nextLine());
} catch (NumberFormatException e) {
System.out.println("Invalid input. Please enter a positive integer.");
}
}
return num;
}
}
```
This implementation uses a Scanner object to read input from the user, and provides a menu with six different actions that can be performed on the library object. Each action is implemented as a separate method that reads input from the user as necessary and calls the appropriate method on the library object. The readLine and readPosInt methods are used to read strings and integers from the user, respectively, and handle any validation or error checking that may be required.
If the user types something which is not an integer, then the nextInt method of the scanner will throw an InputMismatchException. In that case the code of your readPosInt method must catch the exception, use System.out.println to print the error message "You must type an integer!" to the user (use System.out.println for this, not System.err.println, otherwise you might hit a bug in Eclipse...), use the scanner’s nextLine method to read (and ignore) the wrong input typed by the user of the program (if you do not do this, the wrong input typed by the user will remain in the input stream, and the next time you call the nextInt method again, you will get an InputMismatchException again!), and then do the whole thing again (including printing again the string argument of the readPosInt method) to try to read an integer again (hint: put the whole code of the method inside a while loop).java代码
Sure, I understand your question. Here's a possible implementation of the `readPosInt` method in Java that handles the scenario you described:
```java
import java.util.InputMismatchException;
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
int n = readPosInt("Please enter a positive integer: ");
System.out.println("You entered: " + n);
}
public static int readPosInt(String prompt) {
Scanner scanner = new Scanner(System.in);
int n = 0;
boolean success = false;
while (!success) {
System.out.print(prompt);
try {
n = scanner.nextInt();
if (n > 0) {
success = true;
} else {
System.out.println("You must type a positive integer!");
}
} catch (InputMismatchException e) {
System.out.println("You must type an integer!");
scanner.nextLine(); // consume wrong input
}
}
return n;
}
}
```
In this implementation, we use a `while` loop to keep trying to read an integer until we succeed. Inside the loop, we print the prompt and attempt to read an integer using `scanner.nextInt()`. If this call throws an `InputMismatchException`, we catch it and print the error message "You must type an integer!" to the user using `System.out.println()`. We also use `scanner.nextLine()` to consume the wrong input typed by the user, so that the input stream is properly cleared and the next call to `scanner.nextInt()` doesn't fail again.
If the input is an integer, we check if it's positive and set the `success` flag to `true` if it is. If the input is not positive, we print the error message "You must type a positive integer!" to the user using `System.out.println()`.
Finally, if we succeed in reading a positive integer, we return it from the method.
相关推荐
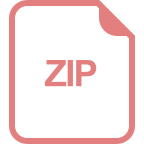
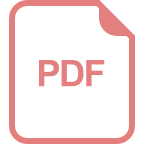












