电力公司的电费计算标准如下:200度以下,以每度0.10元计算。200~500度之间以每30元计算,超过500度,则以0.60元计算。 * 输入本月用电度数,输出本月电费和用电重的之值。 编写一个程序实现该功能,要考虑电费计算过程中程序出现的各种异常,必要时可用自定义异常
时间: 2024-02-28 22:54:43 浏览: 22
好的,以下是实现该程序的Java代码,它考虑了输入数据的异常情况,并使用了自定义异常类ElectricityException:
```java
import java.util.Scanner;
public class ElectricityBill {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
System.out.print("请输入本月用电度数:");
int degree = 0;
try {
degree = sc.nextInt();
if (degree <= 0) {
throw new ElectricityException("用电度数不能小于等于0");
}
} catch (Exception e) {
System.out.println("输入异常:" + e.getMessage());
return;
}
double fee = 0;
if (degree <= 200) {
fee = degree * 0.1;
} else if (degree <= 500) {
fee = (degree - 200) / 30.0 * 1 + 20;
} else {
fee = (degree - 500) * 0.6 + 20 + 10;
}
double weight = degree * 0.25;
System.out.println("本月电费为:" + fee + "元,用电重量为:" + weight + "千克");
}
}
class ElectricityException extends Exception {
public ElectricityException(String message) {
super(message);
}
}
```
程序中先利用Scanner类获取用户输入的电度数degree,并通过try-catch块处理输入异常情况。如果输入的用电度数小于等于0,则抛出自定义异常类ElectricityException。否则,计算电费和用电重量,并输出结果。
由于输入的用电度数可能会出现非法输入,例如输入小数、字符等,因此需要通过try-catch块进行异常处理。如果出现异常,则会输出异常信息并结束程序。
程序中还定义了自定义异常类ElectricityException,用于处理输入用电度数小于等于0的异常情况。
相关推荐
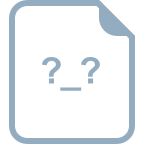














