java文件读写 outputstream,用Java中的多个线程写入FileOutputStream
时间: 2024-05-13 14:16:51 浏览: 7
可以通过创建多个线程并行写入同一个FileOutputStream来实现多线程写入文件。以下是一个简单的示例代码:
```java
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
public class MultiThreadedFileWriter {
public static void main(String[] args) throws Exception {
File file = new File("output.txt");
FileOutputStream fos = new FileOutputStream(file);
Thread thread1 = new Thread(new FileWriterThread(fos, "Thread 1\n"));
Thread thread2 = new Thread(new FileWriterThread(fos, "Thread 2\n"));
Thread thread3 = new Thread(new FileWriterThread(fos, "Thread 3\n"));
thread1.start();
thread2.start();
thread3.start();
thread1.join();
thread2.join();
thread3.join();
fos.close();
}
public static class FileWriterThread implements Runnable {
private final FileOutputStream fos;
private final String data;
public FileWriterThread(FileOutputStream fos, String data) {
this.fos = fos;
this.data = data;
}
@Override
public void run() {
try {
fos.write(data.getBytes());
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
```
在此示例中,我们创建了一个FileOutputStream并将其传递给三个不同的线程,每个线程都会写入不同的数据。我们在每个线程上调用start()方法来启动它们,并在主线程上调用join()方法来等待它们完成。最后,我们关闭FileOutputStream。请注意,同一时间只能有一个线程写入FileOutputStream,因此它们将按顺序排队写入。
相关推荐
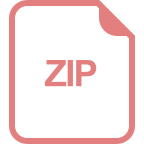
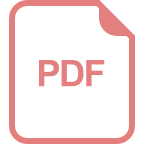














