定义包含学号、姓名和成绩信息的学生类Student。在测试类中定义存放n个学生对象存放到某个集合对象中(具体集合自行确定),然后按成绩进行降序排列,并将排序结果(类Student对象)保存到文件stud.dat中,再读出文件stud.dat中所有的学生信息并显示。
时间: 2024-02-29 11:52:27 浏览: 64
以下是学生类Student的定义:
```cpp
#include <iostream>
#include <string>
#include <fstream>
#include <vector>
#include <algorithm>
using namespace std;
class Student {
private:
int sid;
string name;
double score;
public:
Student(int sid = 0, string name = "", double score = 0.0) {
this->sid = sid;
this->name = name;
this->score = score;
}
int getSid() const {
return sid;
}
string getName() const {
return name;
}
double getScore() const {
return score;
}
void setSid(int sid) {
this->sid = sid;
}
void setName(string name) {
this->name = name;
}
void setScore(double score) {
this->score = score;
}
void display() const {
cout << "学号:" << sid << ",姓名:" << name << ",成绩:" << score << endl;
}
};
```
以下是测试类的实现:
```cpp
int main() {
vector<Student> students;
int n;
cout << "请输入学生人数:";
cin >> n;
for (int i = 0; i < n; i++) {
int sid;
string name;
double score;
cout << "请输入第" << i + 1 << "个学生的学号、姓名、成绩:";
cin >> sid >> name >> score;
Student student(sid, name, score);
students.push_back(student);
}
sort(students.begin(), students.end(), [](const Student& s1, const Student& s2) {
return s1.getScore() > s2.getScore();
});
ofstream fout("stud.dat", ios::binary);
for (const auto& student : students) {
fout.write(reinterpret_cast<const char*>(&student), sizeof(Student));
}
fout.close();
ifstream fin("stud.dat", ios::binary);
vector<Student> sortedStudents;
while (!fin.eof()) {
Student student;
fin.read(reinterpret_cast<char*>(&student), sizeof(Student));
sortedStudents.push_back(student);
}
fin.close();
cout << "按成绩降序排列后的学生信息如下:" << endl;
for (const auto& student : sortedStudents) {
student.display();
}
return 0;
}
```
在测试类中,我们首先用一个vector容器存放n个学生对象,然后按成绩进行降序排列,最后将结果保存到文件stud.dat中。在读取文件时,我们使用了一个while循环来读取所有的学生信息,并将其存放到一个新的vector容器sortedStudents中。最后,我们再输出这个容器中的所有学生信息。
阅读全文
相关推荐
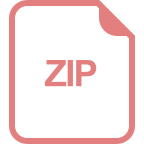
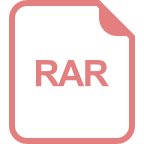
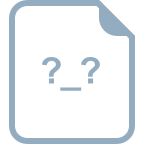






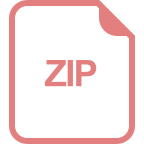
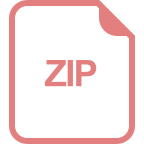
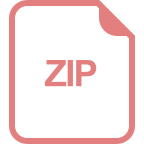
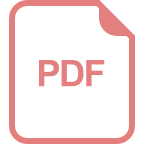
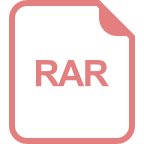
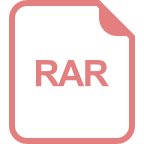
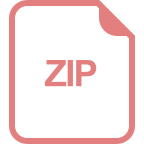
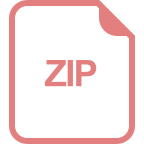
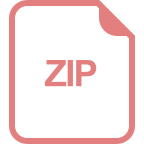
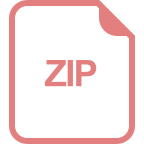