这个错误一般是由pandas无法确定Excel文件的格式所引起的。要解决这个问题,必须手动指定一个引擎来读取Excel文件。
时间: 2023-11-22 13:55:04 浏览: 67
是的,你说得对。pandas的read_excel()函数在读取Excel文件时需要知道文件的格式,一般情况下会自动推断出文件的格式。但是如果文件的格式比较复杂,或者文件名中包含特殊字符,pandas可能无法正确推断出文件格式,此时需要手动指定一个引擎来读取Excel文件。
常见的引擎包括xlrd和openpyxl,可以在read_excel()函数中通过engine参数来指定。例如:
```
import pandas as pd
df = pd.read_excel('file.xlsx', engine='openpyxl')
```
这样就会使用openpyxl引擎来读取Excel文件了。如果还是出现错误,可以尝试更换引擎或者检查文件格式是否正确。
相关问题
报错结果如下Traceback (most recent call last): File "/Users/zhengyaqi/PycharmProjects/pythonProject13/main.py", line 32, in <module> find_and_copy_files(source_folder, target_folder, excel_file) File "/Users/zhengyaqi/PycharmProjects/pythonProject13/main.py", line 18, in find_and_copy_files file_data = pd.read_excel(file_path) File "/Users/zhengyaqi/opt/anaconda3/envs/py/lib/python3.10/site-packages/pandas/io/excel/_base.py", line 478, in read_excel io = ExcelFile(io, storage_options=storage_options, engine=engine) File "/Users/zhengyaqi/opt/anaconda3/envs/py/lib/python3.10/site-packages/pandas/io/excel/_base.py", line 1500, in __init__ raise ValueError( ValueError: Excel file format cannot be determined, you must specify an engine manually.
这个错误是由于`pandas`无法确定 Excel 文件的格式引起的。为了解决这个问题,你可以手动指定一个引擎来读取 Excel 文件。常用的引擎包括 `xlrd`、`openpyxl` 和 `pyxlsb`。
在 `pd.read_excel()` 方法中,你可以添加一个 `engine` 参数来指定引擎。例如,如果你安装了 `xlrd` 引擎,你可以将代码修改为:
```python
file_data = pd.read_excel(file_path, engine='xlrd')
```
如果没有安装 `xlrd`,你可以尝试使用其他可用的引擎,例如 `openpyxl`:
```python
file_data = pd.read_excel(file_path, engine='openpyxl')
```
根据你的实际情况修改代码并选择适合的引擎。如果你不确定应该使用哪个引擎,可以尝试安装 `xlrd`:
```
pip install xlrd
```
然后在代码中使用 `engine='xlrd'` 参数。
记得导入所需的库,例如:
```python
import os
import shutil
import pandas as pd
```
希望这可以解决你的问题!如果还有其他疑问,请随时向我询问。
运行以上代码出现ValueError: Excel file format cannot be determined, you must specify an engine manually. 如何解决?
这个错误通常是由于pandas无法自动确定Excel文件的格式引起的。您可以尝试手动指定引擎来解决此问题。请使用以下修改后的代码:
```python
import pandas as pd
# 将xls文件转为xlsx格式
xls_file = 'input_file.xls'
xlsx_file = 'temp_file.xlsx'
df = pd.read_excel(xls_file, engine='xlrd') # 指定引擎为'xlrd'
df.to_excel(xlsx_file, index=False)
# 读取xlsx文件
df = pd.read_excel(xlsx_file)
# 对第一列第二行以后的数字进行排序,并保持行数据跟随
df.iloc[:, 1:] = df.iloc[:, 1:].apply(lambda x: sorted(x), axis=1)
# 创建替换字典
replace_dict = {
'10001': '平面度',
'20001': '平面度',
'30001': '平面度',
'40001': '平面度',
'50001': '平面度',
'60001': '平面度',
'70001': '平面度',
'80001': '平面度',
'90001': '平面度'
}
# 替换数值
df = df.replace(replace_dict)
# 保存修改后的CSV文件
csv_file = 'output_file.csv'
df.to_csv(csv_file, index=False)
```
在代码中,通过在`read_excel`函数中添加`engine='xlrd'`来手动指定使用'xlrd'引擎进行解析。这样应该可以解决`ValueError: Excel file format cannot be determined, you must specify an engine manually.`的问题。请确保您已经安装了`xlrd`库,如果没有安装,可以使用`!pip install xlrd`命令进行安装。
阅读全文
相关推荐
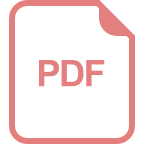
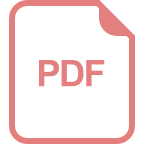
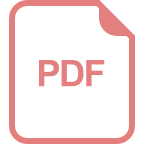
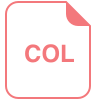
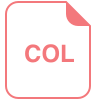
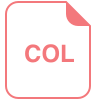
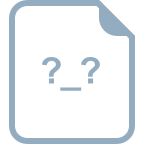
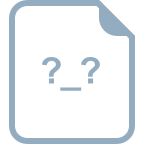
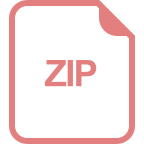
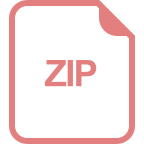
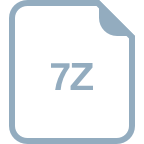