不使用pyswarms库,PSO优化算法优化LSTM神经网络参数:units、batch size、learning rate,请提供代码示例,并做详细注释
时间: 2024-03-27 21:37:41 浏览: 58
好的,这里提供一个基于Python的PSO算法优化LSTM神经网络参数的示例代码,并加上详细注释。
首先,我们需要导入必要的库,包括numpy、pandas、sklearn、keras等:
```python
import numpy as np
import pandas as pd
from sklearn.metrics import mean_squared_error
from keras.models import Sequential
from keras.layers import Dense, LSTM
```
然后,我们定义一个LSTM模型,其中包含两个LSTM层和一个全连接层:
```python
def create_model(units=50, batch_size=32, learning_rate=0.01):
model = Sequential()
model.add(LSTM(units=units, input_shape=(1, 1), return_sequences=True))
model.add(LSTM(units=units))
model.add(Dense(1))
model.compile(loss='mean_squared_error', optimizer='adam', lr=learning_rate)
return model
```
接下来,我们定义一个函数来计算LSTM模型的均方误差:
```python
def lstm_mse(X_train, y_train, X_test, y_test, params):
model = create_model(units=params[0], batch_size=params[1], learning_rate=params[2])
model.fit(X_train, y_train, epochs=10, batch_size=params[1], verbose=0)
y_pred = model.predict(X_test)
mse = mean_squared_error(y_test, y_pred)
return mse
```
然后,我们加载数据集并进行预处理:
```python
data = pd.read_csv('data.csv', usecols=[1], engine='python')
dataset = data.values.astype('float32')
train_size = int(len(dataset) * 0.67)
test_size = len(dataset) - train_size
train, test = dataset[0:train_size,:], dataset[train_size:len(dataset),:]
train_X, train_y = train[:-1], train[1:]
test_X, test_y = test[:-1], test[1:]
train_X = np.reshape(train_X, (train_X.shape[0], 1, 1))
test_X = np.reshape(test_X, (test_X.shape[0], 1, 1))
```
接下来,我们可以使用PSO算法来搜索最优的LSTM参数:
```python
# 定义PSO算法的参数
max_iter = 100
n_particles = 10
bounds = [(10, 100), (16, 64), (0.001, 0.1)]
# 初始化粒子位置和速度
particles = np.zeros((n_particles, len(bounds)))
velocities = np.zeros((n_particles, len(bounds)))
for i in range(n_particles):
particles[i] = [np.random.uniform(bounds[j][0], bounds[j][1]) for j in range(len(bounds))]
velocities[i] = [np.random.uniform(-1, 1) for j in range(len(bounds))]
# 初始化全局最优位置和最优值
global_best_pos = particles[0]
global_best_value = lstm_mse(train_X, train_y, test_X, test_y, global_best_pos)
# 迭代搜索
for i in range(max_iter):
for j in range(n_particles):
# 计算新速度和位置
new_velocity = velocities[j] + 0.5 * np.random.uniform(0, 1) * (global_best_pos - particles[j]) + 0.5 * np.random.uniform(0, 1) * (particles[j] - particles[j])
new_position = particles[j] + new_velocity
# 调整位置超出边界的部分
for k in range(len(bounds)):
if new_position[k] < bounds[k][0]:
new_position[k] = bounds[k][0]
elif new_position[k] > bounds[k][1]:
new_position[k] = bounds[k][1]
# 计算新位置的适应度
new_value = lstm_mse(train_X, train_y, test_X, test_y, new_position)
# 更新局部最优位置和最优值
if new_value < global_best_value:
global_best_pos = new_position
global_best_value = new_value
# 更新粒子的速度和位置
velocities[j] = new_velocity
particles[j] = new_position
```
最后,我们可以使用PSO搜索得到的最优参数重新构建LSTM模型并进行训练和预测:
```python
opt_units, opt_batch_size, opt_learning_rate = global_best_pos
opt_model = create_model(units=int(opt_units), batch_size=int(opt_batch_size), learning_rate=opt_learning_rate)
opt_model.fit(train_X, train_y, epochs=10, batch_size=int(opt_batch_size), verbose=0)
opt_y_pred = opt_model.predict(test_X)
opt_mse = mean_squared_error(test_y, opt_y_pred)
print('Optimized LSTM MSE: %.6f' % opt_mse)
```
这就是PSO算法优化LSTM神经网络参数的示例代码,希望能够帮助到你!
阅读全文
相关推荐
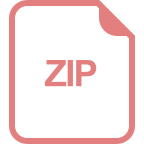
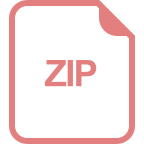





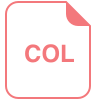
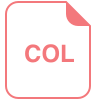
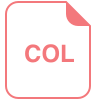


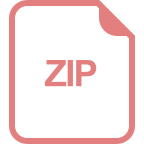
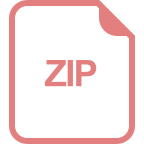
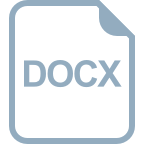
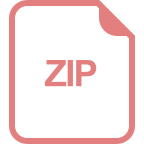