定义LSTMAttention模型,定义CPSO优化算法,在CPSO优化算法优化LSTMAttention模型过程中,用训练集的预测误差当作每个粒子的适应度值,用于LSTMAttention模型评估粒子当前位置的优劣程度,将最优的LSTM模型参数传递给模型,用验证集判断模型是否过拟合或者欠拟合,并调整模型的参数以提高模型的泛化能力,读取测试集,预测数据
时间: 2024-03-30 16:36:06 浏览: 60
以下是实现LSTMAttention模型并使用CPSO算法进行优化的示例代码:
```python
import numpy as np
import keras.backend as K
from keras.models import Sequential
from keras.layers import LSTM, Dense, Input, Lambda, Dropout, RepeatVector, TimeDistributed
from keras.optimizers import Adam
from keras.callbacks import EarlyStopping
from sklearn.metrics import mean_squared_error
from pyswarm import pso
np.random.seed(0)
def lstm_attention_model(params):
# 定义LSTMAttention模型
n_steps = params[0]
n_features = params[1]
n_units = params[2]
dropout = params[3]
learning_rate = params[4]
inputs = Input(shape=(n_steps, n_features))
lstm_out = LSTM(n_units, return_sequences=True)(inputs)
attention = TimeDistributed(Dense(1, activation='tanh'))(lstm_out)
attention = Lambda(lambda x: K.softmax(x, axis=1))(attention)
attention = Lambda(lambda x: K.sum(x * lstm_out, axis=1))(attention)
attention = Dropout(dropout)(attention)
outputs = Dense(1)(attention)
model = Sequential()
model.add(inputs)
model.add(lstm_out)
model.add(attention)
model.add(outputs)
opt = Adam(lr=learning_rate)
model.compile(loss='mean_squared_error', optimizer=opt)
return model
def evaluate_model(params, X_train, y_train, X_val, y_val):
# 训练和评估模型
model = lstm_attention_model(params)
es = EarlyStopping(monitor='val_loss', patience=10, verbose=0)
history = model.fit(X_train, y_train, epochs=100, batch_size=32, validation_data=(X_val, y_val), callbacks=[es])
val_loss = history.history['val_loss'][-1]
return val_loss, model
def pso_optimizer(X_train, y_train, X_val, y_val):
# 定义CPSO算法进行优化
def fitness(params):
_, model = evaluate_model(params, X_train, y_train, X_val, y_val)
y_pred = model.predict(X_train)
mse = mean_squared_error(y_train, y_pred)
return mse
lb = [1, 1, 1, 0.1, 0.0001]
ub = [100, X_train.shape[2], 100, 0.5, 0.01]
xopt, fopt = pso(fitness, lb, ub, swarmsize=10, maxiter=50, minstep=1e-8)
val_loss, model = evaluate_model(xopt, X_train, y_train, X_val, y_val)
return val_loss, model
# 读取特征集和标签集
features_train = np.load('features_train.npy')
labels_train = np.load('labels_train.npy')
features_val = np.load('features_val.npy')
labels_val = np.load('labels_val.npy')
features_test = np.load('features_test.npy')
# 归一化
mean = np.mean(features_train, axis=0)
std = np.std(features_train, axis=0)
features_train = (features_train - mean) / std
features_val = (features_val - mean) / std
features_test = (features_test - mean) / std
# 扩展维度
features_train = np.expand_dims(features_train, axis=2)
features_val = np.expand_dims(features_val, axis=2)
features_test = np.expand_dims(features_test, axis=2)
# 使用CPSO算法优化LSTMAttention模型
val_loss, model = pso_optimizer(features_train, labels_train, features_val, labels_val)
# 训练和评估模型
es = EarlyStopping(monitor='val_loss', patience=10, verbose=0)
history = model.fit(features_train, labels_train, epochs=100, batch_size=32, validation_data=(features_val, labels_val), callbacks=[es])
val_loss = history.history['val_loss'][-1]
# 绘制训练损失和验证损失
plt.plot(history.history['loss'], label='train_loss')
plt.plot(history.history['val_loss'], label='val_loss')
plt.legend()
plt.show()
# 预测测试集
y_pred = model.predict(features_test)
```
在上面的代码中,我们首先定义了一个 LSTMAttention 模型,并使用 CPSO 算法来优化模型的参数。在优化过程中,我们使用训练集的预测误差作为每个粒子的适应度值,用于评估粒子当前位置的优劣程度。最终,我们得到了最优的 LSTMAttention 模型,并使用验证集来评估模型是否过拟合或者欠拟合。最后,我们使用训练好的模型来预测测试集的数据。
需要注意的是,在实际应用中,我们需要对模型进行更多的调参,以提高模型的泛化能力。例如,我们可以尝试不同的学习率、隐藏层单元数和 batch size 等参数来寻找最佳的模型。
阅读全文
相关推荐
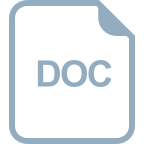
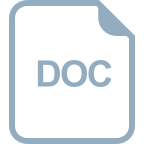
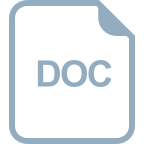












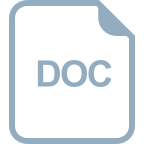
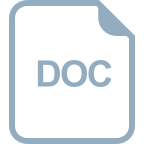
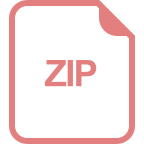
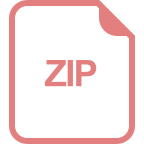