import cv2 import numpy as np def CannyThreshold(lowThreshold): detected_edges = cv2.GaussianBlur(gray,(3,3),0) detected_edges = cv2.Canny(detected_edges,lowThreshold,lowThreshold*ratio,apertureSize = kernel_size) dst = cv2.bitwise_and(img,img,mask = detected_edges) # just add some colours to edges from original image. cv2.imshow('canny demo',dst) lowThreshold = 0 max_lowThreshold = 300 #最大阈值 ratio = 3 kernel_size = 3 #img = cv2.imread('AAA.jpg', cv2.IMREAD_GRAYSCALE) #注释此行图片不灰度化 img = cv2.imread("D:\xiao.jpg") #注释此行上行代码不注释图片灰度化 gray = cv2.imread("D:\xiao.jpg", cv2.IMREAD_GRAYSCALE) cv2.namedWindow('canny demo') cv2.createTrackbar('Min threshold','canny demo',lowThreshold, max_lowThreshold, CannyThreshold) CannyThreshold(0) # initialization if cv2.waitKey(0) == 27: cv2.destroyAllWindows()
时间: 2024-04-01 16:34:36 浏览: 126
这段代码是使用OpenCV库进行Canny边缘检测的操作,并且使用了滑动条来调节最小阈值。其中,首先对输入的图像进行了高斯模糊处理,然后使用Canny函数进行边缘检测,最后使用bitwise_and函数将原图像和检测出的边缘进行合并并显示出来。滑动条的回调函数是CannyThreshold,用于实时更新最小阈值并重新进行边缘检测和显示。如果按下ESC键,则关闭窗口。
阅读全文
相关推荐
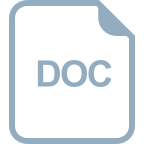
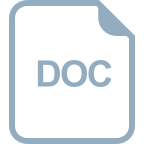
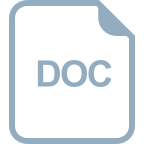
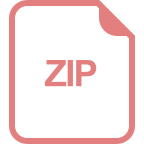
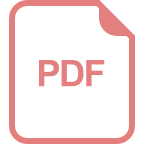
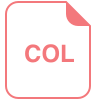
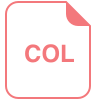
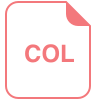
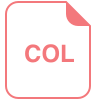
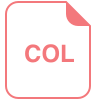
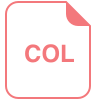
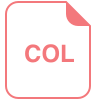
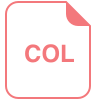
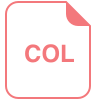
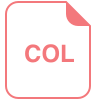
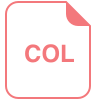
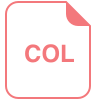
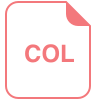
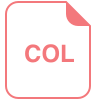