python协程asyncio代码
时间: 2023-09-13 10:14:05 浏览: 52
以下是一个简单的Python协程asyncio代码示例:
```python
import asyncio
async def say_hello():
print("Hello")
await asyncio.sleep(1) # 模拟网络延迟
print("World")
async def main():
await asyncio.gather(say_hello(), say_hello(), say_hello())
asyncio.run(main())
```
在这个例子中,我们定义了两个协程函数:`say_hello` 和 `main`。`say_hello` 打印 "Hello",等待1秒钟模拟网络延迟,然后打印 "World"。`main` 函数使用 `asyncio.gather` 并行运行三个 `say_hello` 协程。
在运行 `main` 函数时,我们使用 `asyncio.run` 运行事件循环,并等待所有协程完成。输出应该如下:
```
Hello
Hello
Hello
World
World
World
```
相关问题
python 协程asyncio爬虫
嗨!关于使用Python协程和asyncio库进行爬虫,我可以给你一些指导。首先,你可以使用asyncio库来实现异步的网络请求和处理。
下面是一个简单的示例代码,使用asyncio和aiohttp库来实现一个基本的爬虫:
```python
import asyncio
import aiohttp
async def fetch(session, url):
async with session.get(url) as response:
return await response.text()
async def main():
urls = [
'http://example.com/page1',
'http://example.com/page2',
'http://example.com/page3'
]
async with aiohttp.ClientSession() as session:
tasks = [fetch(session, url) for url in urls]
results = await asyncio.gather(*tasks)
for result in results:
print(result)
loop = asyncio.get_event_loop()
loop.run_until_complete(main())
```
在这个示例中,我们定义了一个`fetch`函数,用于发送HTTP GET请求并返回响应内容。然后,在`main`函数中,我们定义了要爬取的URL列表,并使用`asyncio.gather`来并发地进行网络请求。
注意,这只是一个简单的示例,实际的爬虫可能需要更复杂的逻辑和处理。你可以根据自己的需求对代码进行修改和扩展。
希望这可以帮助你开始使用asyncio进行爬虫开发!如果还有其他问题,请随时提问。
python协程asyncio爬虫
使用Python的异步编程库asyncio可以实现爬虫功能。在编写爬虫应用时,可以使用异步编程来提升性能。通过使用支持异步编程的aiohttp模块,可以发送异步请求并获取目标数据。
下面是一个示例代码,展示了如何使用asyncio和aiohttp实现一个简单的爬虫:
```python
import aiohttp
import asyncio
async def fetch(session, url):
print("发送请求:", url)
async with session.get(url, verify_ssl=False) as response:
text = await response.text()
print("得到结果:", url, len(text))
async def main():
async with aiohttp.ClientSession() as session:
url_list = [
'https://python.org',
'https://www.baidu.com',
'https://www.pythonav.com'
]
tasks = [asyncio.create_task(fetch(session, url)) for url in url_list]
await asyncio.wait(tasks)
if __name__ == '__main__':
asyncio.run(main())
```
以上代码中,`fetch`函数异步发送请求并获取结果。`main`函数创建了一个`ClientSession`对象,并通过`create_task`函数创建了多个异步任务。通过调用`await asyncio.wait(tasks)`等待所有异步任务完成,实现了并发的请求。
相关推荐
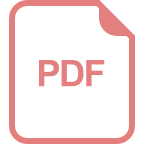
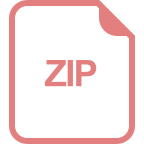
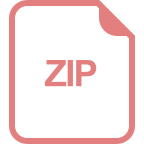












