写一段爬虫代码爬取网页
时间: 2024-03-28 16:18:46 浏览: 29
这里提供一个使用 Python 编写的简单爬虫示例。它可以使用 requests 库来获取网页内容,使用 BeautifulSoup 库来解析 HTML 文档。
```python
import requests
from bs4 import BeautifulSoup
# 需要爬取的网页 URL
url = 'https://www.example.com'
# 使用 requests 库获取网页内容
response = requests.get(url)
# 解析网页内容
soup = BeautifulSoup(response.content, 'html.parser')
# 获取标题
title = soup.title.string
# 输出标题
print(title)
```
上述代码首先使用 requests 库获取网页内容,并将其存储在 `response` 对象中。然后,使用 BeautifulSoup 库将 HTML 文档解析为 Python 对象,并将其存储在 `soup` 对象中。最后,从 `soup` 对象中获取标题,并将其存储在 `title` 变量中。最终,程序输出标题。
相关问题
写一段python爬虫代码爬取网页图片
以下是一个简单的Python爬虫代码,可以爬取指定网页的图片并将其保存到本地:
```python
import requests
from bs4 import BeautifulSoup
import os
url = 'https://example.com' # 指定要爬取的网页
res = requests.get(url)
soup = BeautifulSoup(res.text, 'html.parser')
# 查找所有图片标签
img_tags = soup.find_all('img')
# 遍历所有图片标签,下载图片并保存到本地
for img_tag in img_tags:
img_url = img_tag.get('src')
img_name = img_url.split('/')[-1] # 图片名称为url中最后一个斜杠后的字符串
img_path = os.path.join('./images', img_name) # 保存到当前目录下的images文件夹中
img_res = requests.get(img_url)
with open(img_path, 'wb') as f:
f.write(img_res.content)
print(f'Saved {img_name} successfully.')
```
请注意,此代码仅供参考,实际使用时需要根据具体情况进行修改和优化。同时,爬取网页内容可能涉及法律风险,请谨慎使用。
写一段爬虫代码爬取数据保存为world文档
好的,以下是一个简单的Python爬虫代码,用于爬取指定网站的数据,并将数据保存为world文档。
```Python
import requests
from bs4 import BeautifulSoup
from docx import Document
from docx.shared import Inches
# 爬取网站的URL
url = 'https://www.example.com'
# 发送请求,获取HTML页面
response = requests.get(url)
html = response.content
# 使用BeautifulSoup解析HTML页面
soup = BeautifulSoup(html, 'html.parser')
# 提取需要的数据
data = soup.find('div', {'class': 'content'}).text
# 创建word文档
document = Document()
# 添加标题
document.add_heading('爬取的数据', 0)
# 添加正文
document.add_paragraph(data)
# 保存word文档
document.save('data.docx')
```
在上面的代码中,我们首先使用requests库发送请求,获取目标网站的HTML页面。然后,使用BeautifulSoup解析HTML页面,提取需要的数据。接着,使用python-docx库创建word文档,将数据添加到文档中,最后保存为world文档。您只需要将代码中的url替换为您要爬取的网站,将data替换为您要保存的数据即可。
相关推荐
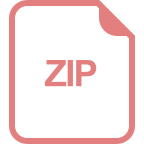











