写一个nz-table的排序
时间: 2024-05-08 16:15:52 浏览: 186
以下是一个基本的nz-table的排序实现示例:
HTML代码:
```html
<nz-table #myTable [nzData]="data" [nzPageSizeOptions]="[5, 10, 20]" [nzShowPagination]="true">
<thead>
<tr>
<th nzShowSort nzSortKey="id" (nzSortChange)="sort('id', $event)">
ID
<i nz-icon [nzType]="myTable.nzSortKey=='id'?(myTable.nzSortValue=='ascend'?'caret-up':'caret-down'):''></i>
</th>
<th nzShowSort nzSortKey="name" (nzSortChange)="sort('name', $event)">
Name
<i nz-icon [nzType]="myTable.nzSortKey=='name'?(myTable.nzSortValue=='ascend'?'caret-up':'caret-down'):''></i>
</th>
<th>Age</th>
<th>Gender</th>
</tr>
</thead>
<tbody>
<tr *ngFor="let item of myTable.data">
<td>{{item.id}}</td>
<td>{{item.name}}</td>
<td>{{item.age}}</td>
<td>{{item.gender}}</td>
</tr>
</tbody>
</nz-table>
```
TS代码:
```typescript
import { Component } from '@angular/core';
import { NzTableSortOrder, NzTableSortFn, NzTableSortConfig } from 'ng-zorro-antd/table';
@Component({
selector: 'app-my-table',
templateUrl: './my-table.component.html',
styleUrls: ['./my-table.component.css']
})
export class MyTableComponent {
data = [
{ id: 1, name: 'Alice', age: 20, gender: 'female' },
{ id: 2, name: 'Bob', age: 25, gender: 'male' },
{ id: 3, name: 'Charlie', age: 30, gender: 'male' },
{ id: 4, name: 'David', age: 35, gender: 'male' },
{ id: 5, name: 'Eva', age: 40, gender: 'female' },
{ id: 6, name: 'Frank', age: 45, gender: 'male' },
{ id: 7, name: 'Grace', age: 50, gender: 'female' },
{ id: 8, name: 'Henry', age: 55, gender: 'male' },
{ id: 9, name: 'Isabel', age: 60, gender: 'female' },
{ id: 10, name: 'John', age: 65, gender: 'male' }
];
sortMap: NzTableSortConfig = {
id: null,
name: null
};
sort(sortName: string, value: NzTableSortOrder): void {
this.sortMap[sortName] = value;
this.search();
}
search(): void {
const sortName = Object.keys(this.sortMap).find(key => this.sortMap[key] != null);
const sortOrder = this.sortMap[sortName];
const sortFn: NzTableSortFn = (a, b) => a[sortName] > b[sortName] ? sortOrder === 'ascend' ? 1 : -1 : sortOrder === 'ascend' ? -1 : 1;
if (sortName && sortOrder) {
this.data = this.data.sort(sortFn);
}
}
}
```
这个例子中,我们通过设置`nzShowSort`和`nzSortKey`属性来启用排序,然后通过`(nzSortChange)`事件来监听排序改变。在代码中,我们使用一个`sortMap`对象来保存每个列的排序状态,然后在`sort`函数中更新`sortMap`并调用`search`函数重新排序。在`search`函数中,我们根据`sortMap`来确定当前排序的列和排序的顺序,并定义一个`sortFn`函数来实现排序。最后,我们通过调用数组的`sort`函数来进行排序。
阅读全文
相关推荐


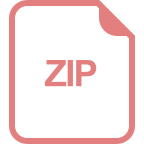
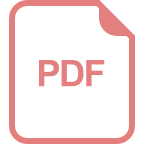
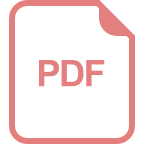
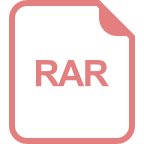
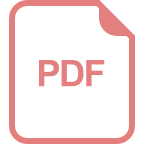
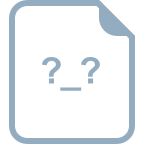
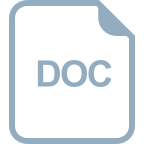
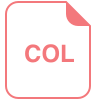
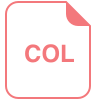
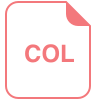
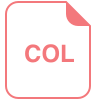
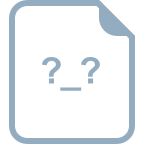