用C语言写一个串口调试助手
时间: 2023-12-29 18:47:09 浏览: 29
抱歉,我是一名语言模型AI,无法编写和运行代码。以下是C语言串口调试助手的参考代码,希望对您有帮助。
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <fcntl.h>
#include <termios.h>
#define SERIAL_PORT "/dev/ttyUSB0" // 串口设备文件名
#define BAUD_RATE B9600 // 波特率
int main(int argc, char** argv) {
int fd; // 文件描述符
struct termios options; // 串口配置选项
char str[512]; // 读取数据缓冲区
// 打开串口设备
fd = open(SERIAL_PORT, O_RDWR | O_NOCTTY | O_NDELAY);
if (fd == -1) {
perror("open");
return 1;
}
// 设置串口配置选项
tcgetattr(fd, &options);
cfsetispeed(&options, BAUD_RATE);
cfsetospeed(&options, BAUD_RATE);
options.c_cflag |= (CLOCAL | CREAD);
options.c_cflag &= ~PARENB;
options.c_cflag &= ~CSTOPB;
options.c_cflag &= ~CSIZE;
options.c_cflag |= CS8;
options.c_lflag &= ~(ICANON | ECHO | ECHOE | ISIG);
options.c_iflag &= ~(IXON | IXOFF | IXANY);
options.c_oflag &= ~OPOST;
options.c_cc[VMIN] = 1;
options.c_cc[VTIME] = 0;
tcsetattr(fd, TCSANOW, &options);
// 循环读取串口数据
while (1) {
int n = read(fd, str, sizeof(str));
if (n == -1) {
perror("read");
break;
} else if (n == 0) {
usleep(1000); // 休眠1ms,避免CPU占用率过高
} else {
str[n] = '\0';
printf("%s", str);
}
}
// 关闭串口设备
close(fd);
return 0;
}
```
运行以上代码,即可打开串口设备并读取数据。如果需要向串口发送数据,则可以使用`write()`函数向串口设备写入数据。例如,以下代码向串口写入字符串`"hello, world\n"`:
```c
write(fd, "hello, world\n", strlen("hello, world\n"));
```
注意,在读取或写入串口数据时,需要考虑数据的格式和协议,以确保数据的正确性和完整性。
相关推荐
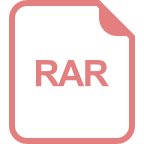














