with open('source.txt','r') as f: f1=f.read() b=open('dest.txt','w+') b.write(f1)
时间: 2023-07-15 16:13:53 浏览: 52
这段代码的功能是将一个名为source.txt的文件中的内容读取出来,然后将读取到的内容写入到一个名为dest.txt的文件中。具体来说,代码首先使用了with语句来打开source.txt文件,并以只读方式读取文件中的内容,读取到的内容会保存在变量f1中。接着,代码使用open函数来打开dest.txt文件,并以写入和读取方式(w+)打开该文件。然后,代码使用write函数将变量f1中的内容写入到dest.txt文件中。最终,代码会关闭source.txt和dest.txt两个文件。
相关问题
from Crypto.Util.number import * f = open('flag.txt', 'r') flag = f.read() f.close() assert flag[:8] == "Dest0g3{" 这段代码的作用
这段代码的作用是:
1. 导入 `Crypto.Util.number` 模块,该模块提供了一些与数字相关的密码学功能。
2. 打开名为 'flag.txt' 的文件,并将文件内容读取到变量 `flag` 中。
3. 关闭文件。
4. 使用断言语句(`assert`)检查变量 `flag` 的前8个字符是否等于 "Dest0g3{"。如果不相等,将引发异常。
综上所述,这段代码的目的是读取名为 'flag.txt' 的文件,并确保文件内容的前8个字符是 "Dest0g3{"。
python【问题描述】已知文本文件source.txt,请将其中内容复制到dest.txt中。 【输入形式】 【输出形式】 【样例输入】dest.txt文件内容如下:
抱歉,作为语言模型,我无法直接访问您的计算机上的文件系统,也不能进行文件复制操作。但是,您可以在Python中使用以下代码将一个文件的内容复制到另一个文件中:
```python
with open("source.txt", "r") as source:
with open("dest.txt", "w") as dest:
dest.write(source.read())
```
其中,`source.txt`是原始文件名,`dest.txt`是目标文件名。该代码将打开原始文件,读取其内容,并将其写入目标文件。请确保在运行代码之前,您已经将原始文件放置在正确的位置,并且目标文件不存在或者您希望覆盖其内容。
相关推荐
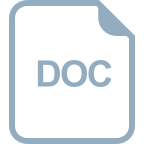
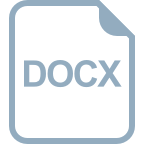
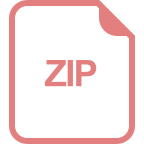
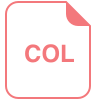
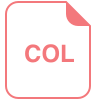
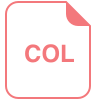
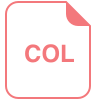









