用c语言编程:写一个函数 days ,实现第1题的计算,由主函数将年、月、日传递给 days 函数,计算后将日子数传回主函数输出。
时间: 2024-02-22 20:00:25 浏览: 31
好的,以下是用C语言编写的代码:
```c
#include <stdio.h>
int isLeapYear(int year) {
return (year % 4 == 0 && year % 100 != 0) || year % 400 == 0;
}
int days(int year, int month, int day) {
int days[] = {0,31,59,90,120,151,181,212,243,273,304,334};
int totalDays = days[month - 1] + day;
if (isLeapYear(year) && month > 2) {
totalDays++;
}
return totalDays;
}
int main() {
int year = 2021, month = 8, day = 12;
int totalDays = days(year, month, day);
printf("%d年%d月%d日是%d年的第%d天\n", year, month, day, year, totalDays);
return 0;
}
```
代码解析如下:
- 首先定义了一个 `isLeapYear` 函数,用于判断闰年。如果该年能被4整除但不能被100整除,或者能被400整除,则为闰年。
- 接着定义了一个 `days` 函数,用于计算该日在本年中是第几天。首先定义了一个每个月份累计天数的数组 `days`,然后根据输入的年月日计算出该日期距离该年1月1日的天数,然后根据是否闰年以及当前月份是否大于2月来判断是否需要加上一个闰年的2月29日的天数,并将总天数返回。
- 最后在 `main` 函数中定义了年月日变量 `year`、`month`、`day`,调用 `days` 函数计算出该日期在本年中的天数,并输出结果。
同样需要注意,在实际使用中,可能需要增加一些输入校验和错误处理的代码,以保证程序的健壮性。
相关推荐
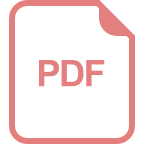
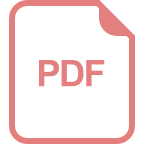














