view.py 中 render 的作用
时间: 2024-05-16 12:19:49 浏览: 133
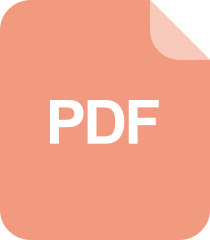
web.py 十分钟创建简易博客实现代码
在 Django 中,render() 是一个快速生成 HTTP 响应的便捷函数,通常用于渲染模板。它的作用是将模板与上下文数据结合起来,生成一个包含上下文数据的 HTML 页面,并返回给客户端。
具体来说,render() 函数接收三个必需参数和一个可选参数:
- request:表示当前的 HTTP 请求对象。
- template_name:表示要使用的模板文件的名称。
- context:表示一个字典,包含要传递给模板的上下文数据。
可选参数 content_type 表示要返回的响应的类型,通常是 "text/html"。如果未指定,则默认为 text/html。
在处理客户端请求时,Django 首先通过 URL 匹配找到对应的视图函数,然后调用该视图函数处理请求,并生成一个 HttpResponse 对象作为响应返回给客户端。render()函数常用于在视图函数中生成 HttpResponse 对象。
例如,在视图函数中调用 render() 函数,将生成一个包含模板渲染结果的 HttpResponse 对象,并将其返回给客户端,从而呈现一个 HTML 页面。
阅读全文
相关推荐
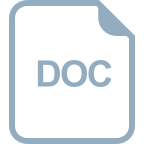
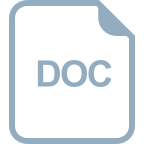
















