用javaJFrame写一个背单词程序,要求从文件中读取单词,在文本域中显示出来,可以更改显示的时间
时间: 2024-02-13 19:01:23 浏览: 30
好的,我可以为您提供一个使用 Java Swing 编写的背单词程序的代码,具体实现功能如下:
1. 从文件中读取单词和其对应的意思。
2. 在文本域中显示一个单词,并输出其对应的意思。
3. 用户输入该单词的意思。
4. 程序判断用户输入是否正确,并输出相应信息。
5. 程序自动切换到下一个单词,并等待一定时间后显示下一个单词。
6. 用户可以通过修改程序中的时间间隔来更改显示时间。
7. 程序循环执行,直到用户选择退出。
以下是代码实现:
```java
import java.awt.*;
import java.awt.event.*;
import java.io.*;
import java.util.*;
import javax.swing.*;
public class VocabularyQuiz extends JFrame implements ActionListener {
private Map<String, String> vocabulary;
private List<String> keys;
private int currentIndex;
private JLabel wordLabel;
private JTextArea meaningTextArea;
private JTextField answerTextField;
private JButton submitButton;
private Timer timer;
private int interval;
public VocabularyQuiz() {
super("Vocabulary Quiz");
vocabulary = new HashMap<String, String>();
try {
File file = new File("vocabulary.txt");
Scanner fileInput = new Scanner(file);
while (fileInput.hasNextLine()) {
String line = fileInput.nextLine();
String[] words = line.split(":");
vocabulary.put(words[0].trim(), words[1].trim());
}
fileInput.close();
} catch (FileNotFoundException e) {
JOptionPane.showMessageDialog(this, "File not found!", "Error", JOptionPane.ERROR_MESSAGE);
System.exit(1);
}
keys = new ArrayList<String>(vocabulary.keySet());
Collections.shuffle(keys);
currentIndex = 0;
wordLabel = new JLabel("");
wordLabel.setFont(new Font("Arial", Font.BOLD, 24));
add(wordLabel, BorderLayout.NORTH);
meaningTextArea = new JTextArea("");
meaningTextArea.setEditable(false);
add(new JScrollPane(meaningTextArea), BorderLayout.CENTER);
JPanel answerPanel = new JPanel(new FlowLayout(FlowLayout.CENTER));
answerPanel.add(new JLabel("Answer:"));
answerTextField = new JTextField(20);
answerPanel.add(answerTextField);
submitButton = new JButton("Submit");
submitButton.addActionListener(this);
answerPanel.add(submitButton);
add(answerPanel, BorderLayout.SOUTH);
interval = 5; // default interval is 5 seconds
timer = new Timer(interval * 1000, new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
showNextWord();
}
});
timer.start();
setPreferredSize(new Dimension(400, 300));
pack();
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setLocationRelativeTo(null);
setVisible(true);
}
@Override
public void actionPerformed(ActionEvent e) {
String answer = answerTextField.getText().trim();
if (answer.equalsIgnoreCase(vocabulary.get(keys.get(currentIndex)))) {
JOptionPane.showMessageDialog(this, "Correct!", "Result", JOptionPane.INFORMATION_MESSAGE);
} else {
JOptionPane.showMessageDialog(this, "Incorrect! The correct answer is \"" + vocabulary.get(keys.get(currentIndex)) + "\".", "Result", JOptionPane.ERROR_MESSAGE);
}
answerTextField.setText("");
showNextWord();
}
private void showNextWord() {
if (currentIndex == keys.size()) {
timer.stop();
JOptionPane.showMessageDialog(this, "You have finished all the words!", "Finished", JOptionPane.INFORMATION_MESSAGE);
System.exit(0);
}
String word = keys.get(currentIndex);
wordLabel.setText(word);
meaningTextArea.setText(vocabulary.get(word));
currentIndex++;
}
public static void main(String[] args) {
SwingUtilities.invokeLater(new Runnable() {
@Override
public void run() {
new VocabularyQuiz();
}
});
}
}
```
在上述代码中,我们使用了 Java Swing 中的 `JFrame`、`JLabel`、`JTextArea`、`JTextField` 和 `JButton` 组件来构建用户界面。程序在启动时从文件 `vocabulary.txt` 中读取单词和其对应的意思,并使用 `HashMap` 存储这些单词。然后,程序使用 `Timer` 定时器来自动切换到下一个单词,并等待一定时间后显示下一个单词。用户可以通过修改程序中的 `interval` 变量来更改显示时间。程序还处理了用户输入的单词,判断用户输入是否正确,并输出相应信息。最后,程序在用户完成所有单词后给出提示,并退出程序。
相关推荐
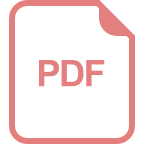














