用java代码写一个背单词程序用到io流和图形化界面
时间: 2023-09-07 14:12:07 浏览: 151
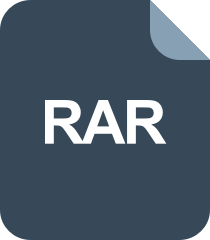
Java背单词程序

好的,下面是一个Java代码示例,实现一个带有IO流和图形化界面的背单词程序:
```java
import java.awt.BorderLayout;
import java.awt.Dimension;
import java.awt.FlowLayout;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.FileWriter;
import java.io.IOException;
import java.util.ArrayList;
import java.util.Collections;
import java.util.Random;
import javax.swing.BorderFactory;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JPanel;
import javax.swing.JTextArea;
import javax.swing.JTextField;
public class Vocabulary extends JFrame implements ActionListener {
private static final long serialVersionUID = 1L;
private ArrayList<String> words;
private ArrayList<String> meanings;
private int currentIndex;
private int correctCount;
private int incorrectCount;
private JTextArea wordArea;
private JTextField meaningField;
private JButton nextButton;
private JButton finishButton;
public Vocabulary() {
super("Vocabulary Quiz");
words = new ArrayList<String>();
meanings = new ArrayList<String>();
currentIndex = 0;
correctCount = 0;
incorrectCount = 0;
JPanel wordPanel = new JPanel(new BorderLayout());
wordPanel.setBorder(BorderFactory.createTitledBorder("Word"));
wordArea = new JTextArea();
wordArea.setEditable(false);
wordArea.setPreferredSize(new Dimension(300, 80));
wordPanel.add(wordArea, BorderLayout.CENTER);
JPanel meaningPanel = new JPanel(new BorderLayout());
meaningPanel.setBorder(BorderFactory.createTitledBorder("Meaning"));
meaningField = new JTextField();
meaningField.setPreferredSize(new Dimension(300, 30));
meaningField.addActionListener(this);
meaningPanel.add(meaningField, BorderLayout.CENTER);
JPanel buttonPanel = new JPanel(new FlowLayout());
nextButton = new JButton("Next");
nextButton.addActionListener(this);
finishButton = new JButton("Finish");
finishButton.addActionListener(this);
buttonPanel.add(nextButton);
buttonPanel.add(finishButton);
JPanel statusPanel = new JPanel(new FlowLayout());
JLabel correctLabel = new JLabel("Correct: 0");
JLabel incorrectLabel = new JLabel("Incorrect: 0");
statusPanel.add(correctLabel);
statusPanel.add(incorrectLabel);
JPanel contentPanel = new JPanel(new BorderLayout());
contentPanel.setBorder(BorderFactory.createEmptyBorder(10, 10, 10, 10));
contentPanel.add(wordPanel, BorderLayout.NORTH);
contentPanel.add(meaningPanel, BorderLayout.CENTER);
contentPanel.add(buttonPanel, BorderLayout.SOUTH);
contentPanel.add(statusPanel, BorderLayout.SOUTH);
add(contentPanel);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setSize(400, 300);
setLocationRelativeTo(null);
setResizable(false);
setVisible(true);
}
public void loadWords(String fileName) {
try {
BufferedReader reader = new BufferedReader(new FileReader(fileName));
String line;
while ((line = reader.readLine()) != null) {
String[] parts = line.split(":");
words.add(parts[0]);
meanings.add(parts[1]);
}
reader.close();
} catch (IOException e) {
e.printStackTrace();
}
}
public void saveResult(String fileName) {
try {
FileWriter writer = new FileWriter(fileName);
writer.write("Correct: " + correctCount + "\n");
writer.write("Incorrect: " + incorrectCount + "\n");
writer.close();
} catch (IOException e) {
e.printStackTrace();
}
}
public void startQuiz() {
if (words.size() == 0) {
return;
}
Collections.shuffle(words, new Random());
showNextWord();
}
private void showNextWord() {
if (currentIndex >= words.size()) {
finishQuiz();
return;
}
wordArea.setText(words.get(currentIndex));
meaningField.setText("");
meaningField.requestFocus();
}
private void finishQuiz() {
saveResult("result.txt");
wordArea.setText("Congratulations! You have finished the quiz.");
meaningField.setEditable(false);
nextButton.setEnabled(false);
finishButton.setEnabled(false);
}
@Override
public void actionPerformed(ActionEvent e) {
if (e.getSource() == meaningField || e.getSource() == nextButton) {
String input = meaningField.getText();
if (input.equalsIgnoreCase(meanings.get(currentIndex))) {
currentIndex++;
correctCount++;
} else {
incorrectCount++;
}
showNextWord();
} else if (e.getSource() == finishButton) {
finishQuiz();
}
}
public static void main(String[] args) {
Vocabulary vocabulary = new Vocabulary();
vocabulary.loadWords("words.txt");
vocabulary.startQuiz();
}
}
```
上述代码中,我们借助了Swing框架实现了一个图形化界面。主窗口包含一个单词显示区域和一个文本框,用户需要在文本框中输入对应的意思。
在`loadWords`方法中,我们通过IO流读取一个文件,将其中的单词和意思添加到列表中。在`saveResult`方法中,我们通过IO流将用户的测试结果保存到一个文件中。
在`startQuiz`方法中,我们将单词列表随机打乱,并调用`showNextWord`方法开始测试。在`showNextWord`方法中,我们会依次显示每个单词,并等待用户输入对应的意思。
在`actionPerformed`方法中,我们会处理用户输入的意思,并根据用户的正确与否更新计数器。如果用户点击“Finish”按钮,测试结束,程序会调用`finishQuiz`方法,显示测试结果并将结果保存到文件中。
在`main`方法中,我们创建了一个`Vocabulary`对象,并调用`loadWords`方法读取单词列表文件,最后调用`startQuiz`方法开始背单词。
阅读全文
相关推荐
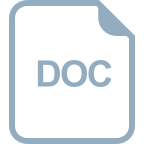
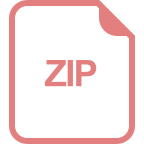
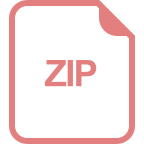
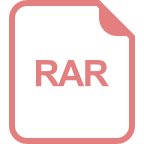
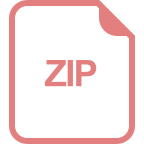
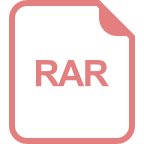
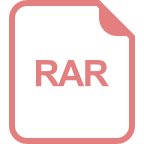
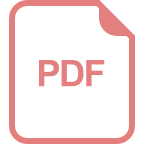
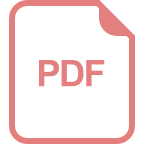
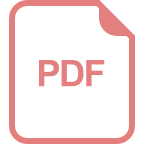
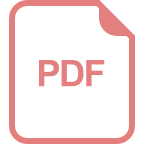
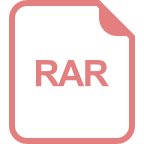