用java的socket来设计一个客户端与服务端通信的图形化界面,图形化用JFrame
时间: 2024-03-07 10:48:23 浏览: 107
好的,我可以为您提供一些关于如何使用Java Socket在JFrame中设计客户端与服务端通信的图形化界面的基本指导。
首先,您需要在客户端和服务端分别实现Java Socket。这可以通过创建一个Socket对象并指定IP地址和端口号来完成。例如,以下是一个简单的客户端Socket实现:
```java
Socket clientSocket = new Socket("localhost", 1234);
```
接下来,在JFrame中创建一个文本框和一个按钮以便用户可以输入消息并将其发送到服务端。您还需要在JFrame中添加一个显示文本的文本区域,以显示从服务端返回的消息。在用户单击“发送”按钮时,客户端应该将消息发送到服务端。
以下是一个简单的客户端JFrame实现:
```java
import java.awt.BorderLayout;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.io.IOException;
import java.io.PrintWriter;
import java.net.Socket;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JPanel;
import javax.swing.JScrollPane;
import javax.swing.JTextArea;
import javax.swing.JTextField;
public class ClientGUI extends JFrame {
private final JTextArea messageArea;
private final JTextField textField;
private final JButton sendButton;
private final Socket clientSocket;
private final PrintWriter out;
public ClientGUI() throws IOException {
super("Chat Client");
// create socket
clientSocket = new Socket("localhost", 1234);
out = new PrintWriter(clientSocket.getOutputStream(), true);
// create UI elements
messageArea = new JTextArea(10, 30);
messageArea.setEditable(false);
JScrollPane scrollPane = new JScrollPane(messageArea);
textField = new JTextField(30);
sendButton = new JButton("Send");
// add action listener to send button
sendButton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
String message = textField.getText();
out.println(message);
textField.setText("");
}
});
// add UI elements to frame
JPanel panel = new JPanel();
panel.add(textField);
panel.add(sendButton);
add(panel, BorderLayout.NORTH);
add(scrollPane, BorderLayout.CENTER);
// set frame properties
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
pack();
setVisible(true);
}
public static void main(String[] args) {
try {
new ClientGUI();
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
在服务端实现中,您需要创建一个ServerSocket并在一个无限循环中等待客户端连接。当客户端连接到服务端时,服务端应该创建一个新的线程来处理客户端的请求。在新线程中,服务端应该接收来自客户端的消息,并将其回显回客户端。
以下是一个简单的服务端实现:
```java
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.net.ServerSocket;
import java.net.Socket;
public class Server {
public static void main(String[] args) throws IOException {
ServerSocket serverSocket = new ServerSocket(1234);
while (true) {
Socket clientSocket = serverSocket.accept();
new Thread(new ClientHandler(clientSocket)).start();
}
}
private static class ClientHandler implements Runnable {
private final Socket clientSocket;
public ClientHandler(Socket clientSocket) {
this.clientSocket = clientSocket;
}
@Override
public void run() {
try {
BufferedReader in = new BufferedReader(
new InputStreamReader(clientSocket.getInputStream()));
String message;
while ((message = in.readLine()) != null) {
System.out.println("Received message: " + message);
clientSocket.getOutputStream().write(message.getBytes());
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
```
希望这些指导对您有所帮助!
阅读全文
相关推荐





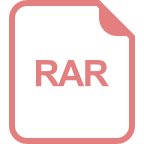






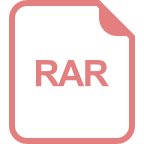



