用c++语言设计一个按时间片轮转发实现处理器调度的程序
时间: 2023-06-20 08:10:42 浏览: 96
下面是一个简单的按时间片轮转调度算法的C++程序:
```c++
#include <iostream>
#include <queue>
#include <vector>
using namespace std;
struct Process {
int pid; // 进程ID
int burst_time; // 执行时间
int remaining_time; // 剩余执行时间
int arrival_time; // 到达时间
int waiting_time; // 等待时间
int turnaround_time; // 周转时间
};
void rr_scheduling(vector<Process>& processes, int time_slice) {
queue<Process*> ready_queue; // 就绪队列
int current_time = 0; // 当前时间
int n = processes.size(); // 进程数
int completed = 0; // 完成进程数
// 将所有进程加入就绪队列
for (int i = 0; i < n; ++i) {
ready_queue.push(&processes[i]);
}
// 循环调度就绪队列中的进程,直到所有进程都执行完毕
while (completed < n) {
Process* current_process = ready_queue.front();
ready_queue.pop();
// 如果当前进程还有剩余执行时间,则执行之
if (current_process->remaining_time > 0) {
int run_time = min(time_slice, current_process->remaining_time);
current_process->remaining_time -= run_time;
current_time += run_time;
// 将在当前时间片之前到达的进程加入就绪队列
for (int i = 0; i < n; ++i) {
if (processes[i].arrival_time <= current_time &&
processes[i].remaining_time > 0 &&
&processes[i] != current_process) {
ready_queue.push(&processes[i]);
}
}
// 如果当前进程执行完毕,则更新其相关时间数据
if (current_process->remaining_time == 0) {
completed++;
current_process->turnaround_time = current_time - current_process->arrival_time;
current_process->waiting_time = current_process->turnaround_time - current_process->burst_time;
cout << "Process " << current_process->pid << " completed. Turnaround time = " << current_process->turnaround_time << ", Waiting time = " << current_process->waiting_time << endl;
}
else {
ready_queue.push(current_process);
}
}
}
}
int main() {
vector<Process> processes = {
{1, 10, 10, 0},
{2, 5, 5, 0},
{3, 8, 8, 0},
{4, 7, 7, 0},
{5, 6, 6, 0}
};
rr_scheduling(processes, 2);
return 0;
}
```
这个程序中,我们定义了一个 `Process` 结构体来表示进程,其中包括进程ID、执行时间、剩余执行时间、到达时间、等待时间和周转时间等信息。
`rr_scheduling` 函数实现了按时间片轮转调度算法。该函数首先将所有进程加入就绪队列,然后循环调度就绪队列中的进程,直到所有进程都执行完毕。对于每个时间片,如果当前进程还有剩余执行时间,则执行之,并将在当前时间片之前到达的进程加入就绪队列。如果当前进程执行完毕,则更新其相关时间数据,并打印出其周转时间和等待时间。
在 `main` 函数中,我们定义了一个测试用的进程向量,并调用 `rr_scheduling` 函数来执行时间片轮转调度算法,时间片大小为2。
相关推荐
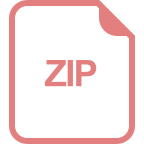
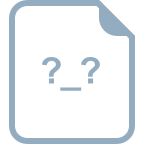














