假设机器人系统通过RS485与某电机控制器通信,采用MODBUS RTU协议。电机控制器内部存放电机电流的起始地址是0x1234(每个地址存在1个字节),电流值占用4个地址(4字节整数,以0.1A为单位,大端格式)。利用功能码3读取该电机控制器内部保存的电机当前电流值。用C语言编写发送03命令和接收数据并处理得到电流值的程序。(不包括通信部分程序)
时间: 2024-03-19 21:45:36 浏览: 158
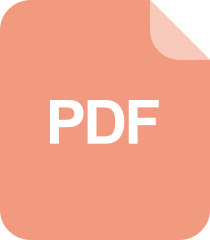
通过采用PLC和变频器相结合实现多电机随动控制系统的设计

好的,以下是C语言代码实现:
```c
#include <stdio.h>
#include <stdint.h>
#include <string.h>
#include <errno.h>
#include <unistd.h>
#include <fcntl.h>
#include <termios.h>
#define START_ADDR 0x1234 // 电机电流的起始地址
#define READ_NUM 4 // 读取4个地址的数据
#define UNIT 0.1 // 电流值单位为0.1A
int main() {
int fd = open("/dev/ttyUSB0", O_RDWR | O_NOCTTY | O_NDELAY); // 串口设备文件描述符
if (fd == -1) {
perror("open");
return -1;
}
struct termios options;
tcgetattr(fd, &options);
options.c_cflag = B9600 | CS8 | CLOCAL | CREAD;
options.c_iflag = IGNPAR;
options.c_oflag = 0;
options.c_lflag = 0;
tcflush(fd, TCIFLUSH);
tcsetattr(fd, TCSANOW, &options);
uint8_t send_buf[8] = {0}; // 发送数据缓冲区
uint8_t recv_buf[8] = {0}; // 接收数据缓冲区
// 发送MODBUS RTU命令
send_buf[0] = 0x01; // 从机地址
send_buf[1] = 0x03; // 功能码
send_buf[2] = (START_ADDR >> 8) & 0xff; // 开始读取的地址高位
send_buf[3] = START_ADDR & 0xff; // 开始读取的地址低位
send_buf[4] = 0x00; // 读取的字节数高位
send_buf[5] = READ_NUM; // 读取的字节数低位
uint16_t crc = 0xFFFF;
for (int i = 0; i < 6; i++) {
crc ^= send_buf[i];
for (int j = 0; j < 8; j++) {
if (crc & 0x0001) {
crc = (crc >> 1) ^ 0xA001;
} else {
crc = crc >> 1;
}
}
}
send_buf[6] = crc & 0xff; // CRC校验低位
send_buf[7] = (crc >> 8) & 0xff; // CRC校验高位
write(fd, send_buf, 8); // 发送数据
// 读取MODBUS RTU响应
int recv_len = 0;
int max_recv_len = 8;
while (recv_len < max_recv_len) {
int len = read(fd, recv_buf + recv_len, max_recv_len - recv_len); // 读取数据
if (len == -1) {
if (errno == EAGAIN) {
printf("timeout\n"); // 超时,退出循环
break;
} else {
perror("read");
return -1;
}
}
recv_len += len;
}
// 解析响应数据
if (recv_len == max_recv_len && recv_buf[0] == 0x01 && recv_buf[1] == 0x03) {
uint8_t *data = recv_buf + 3; // 从第4个字节开始是读取到的数据
uint32_t current_value = ((uint32_t)data[0] << 24) | ((uint32_t)data[1] << 16) | ((uint32_t)data[2] << 8) | data[3]; // 将4字节数据转化为整数
float current = current_value * UNIT; // 计算电流值
printf("Current: %.1fA\n", current);
} else {
printf("Invalid response\n");
}
close(fd);
return 0;
}
```
这段代码实现了通过串口发送MODBUS RTU命令读取电机控制器内部保存的电机当前电流值,并将读取到的电流值打印出来。需要注意的是,该代码没有包括通信部分,因此需要根据实际情况进行修改。
阅读全文
相关推荐
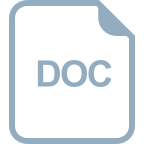
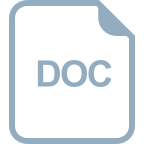

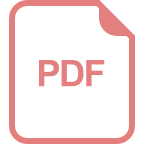
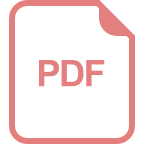
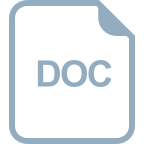
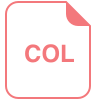
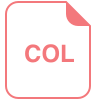
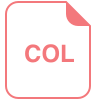
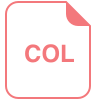
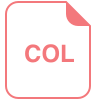
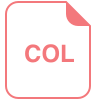
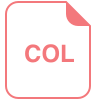
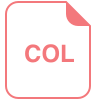
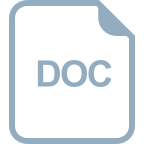
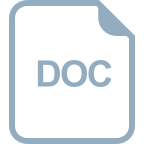