#include <iostream> using namespace std; class class0 { public: virtual ~class0(){} protected: char p; public: char getChar(); }; class class1 : public class0 { public: void printChar(); }; void class1::printChar() { cout << "True" << endl; } int main() { class1 c; c.printChar(); return 0; }
时间: 2024-02-14 16:18:58 浏览: 21
这段代码定义了两个类 `class0` 和 `class1`,其中 `class1` 继承了 `class0`。`class0` 定义了一个受保护的 char 类型的数据成员 `p` 和一个公有的成员函数 `getChar()`,`class1` 定义了一个公有的成员函数 `printChar()`。`class0` 的析构函数是虚函数。
在 `main()` 函数中,创建了一个 `class1` 类型的对象 `c`,然后调用了它的 `printChar()` 成员函数。该函数只是简单地将字符串 "True" 输出到标准输出流中,然后换行。最后返回 0。
需要注意的是,`class0` 的析构函数是虚函数,这意味着当 `class1` 类型的对象被销毁时,会自动调用 `class0` 的析构函数。由于 `class0` 的析构函数是空的,因此这个程序并不会有任何内存泄漏的问题。
相关问题
异常原因#include <iostream> #include<memory> #include<string> using namespace std; class Product { public: virtual void Creat() = 0; }; class ProductA : public Product { public: void Creat() { cout << "创建产品A"; }; }; class ProductB : public Product { public: void Creat() { cout << "创建产品B"; } }; class Factory { public: virtual void makeProduct() = 0; void setProduct(char type_of_product) { if (type_of_product == 'a'){ unique_ptr<Product> productA = make_unique<ProductA>(); this->product = productA.get(); } }; protected: Product* product; }; class Factory1 : public Factory { public: void makeProduct() { cout << "工厂1:"; this->product->Creat(); } }; class Factory2 : public Factory { public: void makeProduct() { cout << "工厂2:"; this->product->Creat(); } }; int main() { unique_ptr<Product> productA = make_unique<ProductA>(); unique_ptr<Factory> factory1 = make_unique<Factory1>(); factory1->setProduct('A'); factory1->makeProduct(); return 0; }
这份代码中存在一些问题:
1. `Factory::setProduct()` 中只有对产品A的处理,没有对产品B的处理。
2. `Factory::product` 指针没有进行初始化,可能会导致程序崩溃。
3. `unique_ptr<Factory> factory1 = make_unique<Factory1>();` 中的 `Factory1` 类没有定义默认构造函数,因此需要手动提供一个构造函数。
以下是修改后的代码:
```
#include <iostream>
#include <memory>
#include <string>
using namespace std;
class Product {
public:
virtual void Creat() = 0;
};
class ProductA : public Product {
public:
void Creat() {
cout << "创建产品A";
};
};
class ProductB : public Product {
public:
void Creat() {
cout << "创建产品B";
}
};
class Factory {
public:
virtual void makeProduct() = 0;
virtual ~Factory() {}
void setProduct(char type_of_product) {
if (type_of_product == 'a') {
unique_ptr<Product> productA = make_unique<ProductA>();
this->product = productA.get();
}
else if (type_of_product == 'b') {
unique_ptr<Product> productB = make_unique<ProductB>();
this->product = productB.get();
}
};
protected:
Product* product = nullptr;
};
class Factory1 : public Factory {
public:
Factory1() {}
void makeProduct() {
cout << "工厂1:";
if (product) {
product->Creat();
}
else {
cout << "未设置产品" << endl;
}
}
};
class Factory2 : public Factory {
public:
Factory2() {}
void makeProduct() {
cout << "工厂2:";
if (product) {
product->Creat();
}
else {
cout << "未设置产品" << endl;
}
}
};
int main() {
unique_ptr<Factory> factory1 = make_unique<Factory1>();
factory1->setProduct('a');
factory1->makeProduct();
return 0;
}
```
修改后的代码中,`Factory::setProduct()` 中加入了对产品B的处理;`Factory::product` 指针进行了初始化;`Factory1` 和 `Factory2` 中分别添加了默认构造函数并且在 `makeProduct()` 中判断是否设置了产品。
优化这段代码并消除bug:#include<iostream> #include<string> using namespace std; class Vehicle{ protected: string name; string color; public: Vehicle(string n,string c):name(n),color(c){}; virtual void display(); }; class Car : public Vehicle{ private: int pas; public: Car(string n,string c,int p):Vehicle(n,c),pas(p){}; void display(){ cout << name << " " << color << " " << pas << endl; }; }; class Truck : public Vehicle{ private: double weight; public: Truck(string n,string c,double d):Vehicle(n,c),weight(d){}; void display(){ cout << name << " " << color << " " << weight << endl; }; }; int main() { Vehicle *p; char type; char name[110],color[110]; int pas; double cap; while(cin>>type) { cin>>name>>color; if(type == 'C') { cin>>pas; Car car(name,color,pas); p = &car; p->display(); } else if(type == 'T') { cin>>cap; Truck truck(name,color,cap); p = &truck; p->display(); } } return 0; }
优化后的代码如下,主要是将类的成员变量改为指针类型,避免在栈上分配内存导致指针失效:
```cpp
#include<iostream>
#include<string>
using namespace std;
class Vehicle{
protected:
string name;
string color;
public:
Vehicle(string n, string c): name(n), color(c){};
virtual void display() = 0;
virtual ~Vehicle(){} // 添加虚析构函数
};
class Car : public Vehicle{
private:
int *pas; // 改为指针类型
public:
Car(string n, string c, int p): Vehicle(n, c){
pas = new int(p); // 动态分配内存
};
void display(){
cout << name << " " << color << " " << *pas << endl;
};
~Car(){ // 添加析构函数,释放内存
delete pas;
}
};
class Truck : public Vehicle{
private:
double *weight; // 改为指针类型
public:
Truck(string n, string c, double d): Vehicle(n, c){
weight = new double(d); // 动态分配内存
};
void display(){
cout << name << " " << color << " " << *weight << endl;
};
~Truck(){ // 添加析构函数,释放内存
delete weight;
}
};
int main() {
Vehicle *p;
char type;
char name[110], color[110];
int pas;
double cap;
while(cin>>type) {
cin>>name>>color;
if(type == 'C') {
cin>>pas;
p = new Car(name, color, pas); // 动态分配内存
p->display();
delete p; // 释放内存
}
else if(type == 'T') {
cin>>cap;
p = new Truck(name, color, cap); // 动态分配内存
p->display();
delete p; // 释放内存
}
}
return 0;
}
```
此外,还需要添加虚析构函数,在子类析构时能够自动调用父类析构函数,释放父类的内存。并且在动态分配内存后需要在适当的时候释放内存,避免内存泄漏。
相关推荐
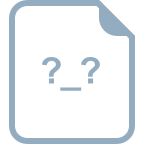













