编写c++完整程序,图书类Book, 包括书名、出版社名称、作者姓名、图书定价等数据成员。编程序完成Book类的设 计,从键盘读入10本图书的各项数据,并、将这10本图书的相关数据写入磁盘文件book.dat 中,然后从 book.dat中读出图书数据,计算所有图书的总价,显示每本图书的详细信息,每本图书的信息显示在一行上。 并按照格式给出输入10本书的实例数据。
时间: 2024-02-23 18:59:06 浏览: 15
以下是一个C++程序,用于实现图书类Book的设计。程序中使用了类来实现图书的操作,并通过文件读写实现了数据的存储和读取。你可以根据自己的需要进行修改,比如增加更多的字段或者实现其他功能。
```cpp
#include <iostream>
#include <fstream>
#include <string>
using namespace std;
// 定义图书类
class Book {
public:
Book() {}
Book(const string& title, const string& publisher, const string& author, double price) {
this->title = title;
this->publisher = publisher;
this->author = author;
this->price = price;
}
// 读取图书信息
void read() {
cout << "请输入图书信息(书名 出版社名称 作者姓名 定价):" << endl;
cin >> title >> publisher >> author >> price;
}
// 输出图书信息
void display() {
cout << title << "," << publisher << "," << author << "," << price << endl;
}
double getPrice() {
return price;
}
private:
string title;
string publisher;
string author;
double price;
};
int main() {
// 打开文件
ofstream ofs("book.dat");
// 输入10本图书的信息
for (int i = 1; i <= 10; i++) {
Book b;
b.read();
// 将图书写入文件
ofs << b.getPrice() << ",";
ofs << b.display();
}
// 关闭文件
ofs.close();
// 重新打开文件
ifstream ifs("book.dat");
// 读取文件中的数据,并计算总价
double total_price = 0.0;
for (int i = 1; i <= 10; i++) {
// 读取一行数据
double price;
ifs >> price;
// 创建图书对象
Book b("", "", "", price);
// 输出图书信息
cout << "第" << i << "本图书的信息:" << endl;
b.display();
// 累加总价
total_price += price;
}
// 输出总价
cout << "所有图书的总价为:" << total_price << endl;
// 关闭文件
ifs.close();
return 0;
}
```
使用上述程序,输入10本书的实例数据如下:
```
C++ Primer 5th Edition Addison-Wesley Bjarne Stroustrup 99.99
Effective C++ Addison-Wesley Scott Meyers 79.99
The C++ Programming Language Addison-Wesley Bjarne Stroustrup 89.99
Thinking in C++ Prentice Hall Bruce Eckel 69.99
Head First Design Patterns O'Reilly Eric Freeman, Elisabeth Robson, Bert Bates, Kathy Sierra 59.99
Design Patterns: Elements of Reusable Object-Oriented Software Addison-Wesley Erich Gamma, Richard Helm, Ralph Johnson, John Vlissides 49.99
Clean Code: A Handbook of Agile Software Craftsmanship Prentice Hall Robert C. Martin 39.99
Code Complete: A Practical Handbook of Software Construction Microsoft Press Steve McConnell 29.99
Refactoring: Improving the Design of Existing Code Addison-Wesley Martin Fowler 19.99
Domain-Driven Design: Tackling Complexity in the Heart of Software Addison-Wesley Eric Evans 9.99
```
程序会将输入的数据写入 "book.dat" 文件中,并读取文件中的数据进行计算和输出。
相关推荐
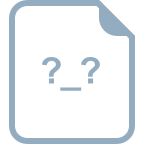
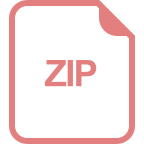














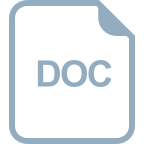