vector<string> 文件读写
时间: 2023-08-13 19:04:37 浏览: 92
要进行文件读写操作,可以使用C++标准库中的`fstream`类。下面是一个示例代码,演示如何使用`fstream`读取和写入一个包含字符串的文件:
```cpp
#include <iostream>
#include <fstream>
#include <vector>
#include <string>
int main() {
std::vector<std::string> data; // 存储读取的字符串数据
// 从文件中读取数据
std::ifstream file("data.txt");
if (file.is_open()) {
std::string line;
while (std::getline(file, line)) {
data.push_back(line);
}
file.close();
std::cout << "文件读取完成!" << std::endl;
} else {
std::cout << "无法打开文件!" << std::endl;
return 1;
}
// 输出读取的数据
std::cout << "文件内容:" << std::endl;
for (const auto& str : data) {
std::cout << str << std::endl;
}
// 向文件中写入数据
std::ofstream outputFile("output.txt");
if (outputFile.is_open()) {
for (const auto& str : data) {
outputFile << str << std::endl;
}
outputFile.close();
std::cout << "文件写入完成!" << std::endl;
} else {
std::cout << "无法创建输出文件!" << std::endl;
return 1;
}
return 0;
}
```
上述代码将从名为"data.txt"的文件中读取数据,并将每一行的字符串存储在一个`vector`容器中。然后,它将输出读取的数据,并将其写入名为"output.txt"的文件中。
请确保在运行代码之前,创建一个名为"data.txt"的文件,并在其中添加一些文本行。
阅读全文
相关推荐
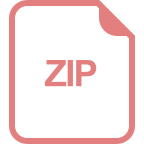








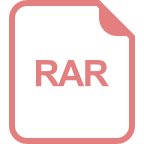
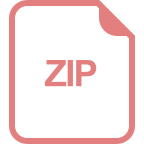
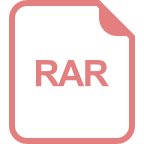
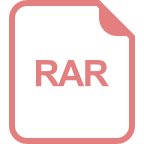
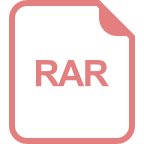
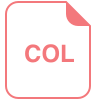
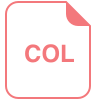
