.net core openssl 加密示例
时间: 2023-08-01 12:09:07 浏览: 173
以下是 .NET Core 中使用 OpenSSL 进行加密的示例代码:
```csharp
using System;
using System.Security.Cryptography;
using System.Text;
using System.Runtime.InteropServices;
public class OpenSSLExample
{
public static void Main()
{
// 加载 OpenSSL 库
const string OPENSSL_LIB = "libcrypto.so.1.1";
IntPtr openssl = LoadLibrary(OPENSSL_LIB);
if (openssl == IntPtr.Zero)
{
Console.WriteLine("无法加载 OpenSSL 库: {0}", OPENSSL_LIB);
return;
}
// 准备密钥和数据
byte[] key = Encoding.UTF8.GetBytes("my secret key");
byte[] plaintext = Encoding.UTF8.GetBytes("hello world");
// 加密数据
byte[] ciphertext = Encrypt(plaintext, key);
// 解密数据
byte[] decryptedtext = Decrypt(ciphertext, key);
// 输出结果
Console.WriteLine("原始数据: {0}", Encoding.UTF8.GetString(plaintext));
Console.WriteLine("加密后的数据: {0}", Convert.ToBase64String(ciphertext));
Console.WriteLine("解密后的数据: {0}", Encoding.UTF8.GetString(decryptedtext));
// 卸载 OpenSSL 库
FreeLibrary(openssl);
}
[DllImport("libdl.so")]
private static extern IntPtr dlopen(string filename, int flags);
[DllImport("libdl.so")]
private static extern IntPtr dlerror();
[DllImport("libdl.so")]
private static extern IntPtr dlsym(IntPtr handle, string symbol);
[DllImport("libdl.so")]
private static extern int dlclose(IntPtr handle);
[DllImport("libc.so.6")]
private static extern int puts(string s);
[DllImport("libc.so.6")]
private static extern int printf(string format, IntPtr arg);
private static IntPtr LoadLibrary(string filename)
{
int RTLD_NOW = 2;
IntPtr handle = dlopen(filename, RTLD_NOW);
if (handle == IntPtr.Zero)
{
IntPtr error = dlerror();
printf("无法加载库: %s\n", error);
}
return handle;
}
private static void FreeLibrary(IntPtr handle)
{
dlclose(handle);
}
private static byte[] Encrypt(byte[] plaintext, byte[] key)
{
IntPtr evp_cipher = dlsym(LoadLibrary("libcrypto.so.1.1"), "EVP_aes_256_cbc");
byte[] iv = GenerateRandomBytes(16);
byte[] ciphertext = new byte[plaintext.Length + 16];
int ciphertext_len;
using (var ctx = new EVP_CIPHER_CTX())
{
EVP_CipherInit_ex(ctx, evp_cipher, IntPtr.Zero, key, iv, 1);
EVP_CipherUpdate(ctx, ciphertext, out ciphertext_len, plaintext, plaintext.Length);
EVP_CipherFinal_ex(ctx, ciphertext, out ciphertext_len);
}
return ciphertext;
}
private static byte[] Decrypt(byte[] ciphertext, byte[] key)
{
IntPtr evp_cipher = dlsym(LoadLibrary("libcrypto.so.1.1"), "EVP_aes_256_cbc");
byte[] iv = new byte[16];
Array.Copy(ciphertext, iv, 16);
byte[] plaintext = new byte[ciphertext.Length - 16];
int plaintext_len;
using (var ctx = new EVP_CIPHER_CTX())
{
EVP_CipherInit_ex(ctx, evp_cipher, IntPtr.Zero, key, iv, 0);
EVP_CipherUpdate(ctx, plaintext, out plaintext_len, ciphertext, 16, ciphertext.Length - 16);
EVP_CipherFinal_ex(ctx, plaintext, out plaintext_len);
}
return plaintext;
}
private static byte[] GenerateRandomBytes(int length)
{
byte[] bytes = new byte[length];
using (var rng = new RNGCryptoServiceProvider())
{
rng.GetBytes(bytes);
}
return bytes;
}
private class EVP_CIPHER_CTX : SafeHandle
{
public EVP_CIPHER_CTX() : base(IntPtr.Zero, true) {}
public override bool IsInvalid
{
get { return handle == IntPtr.Zero; }
}
protected override bool ReleaseHandle()
{
EVP_CIPHER_CTX_free(handle);
return true;
}
[DllImport("libcrypto.so.1.1")]
private static extern IntPtr EVP_CIPHER_CTX_new();
[DllImport("libcrypto.so.1.1")]
private static extern void EVP_CIPHER_CTX_free(IntPtr ctx);
[DllImport("libcrypto.so.1.1")]
private static extern int EVP_CipherInit_ex(EVP_CIPHER_CTX ctx, IntPtr cipher, IntPtr engine, byte[] key, byte[] iv, int enc);
[DllImport("libcrypto.so.1.1")]
private static extern int EVP_CipherUpdate(EVP_CIPHER_CTX ctx, byte[] @out, out int outl, byte[] @in, int inl);
[DllImport("libcrypto.so.1.1")]
private static extern int EVP_CipherFinal_ex(EVP_CIPHER_CTX ctx, byte[] @out, out int outl);
}
}
```
在上面的示例中,我们使用 OpenSSL 库中的 AES-256-CBC 算法对数据进行加密和解密,可以根据需要修改算法和密钥长度。注意,需要在 Linux 环境下编译和运行此示例。
阅读全文
相关推荐
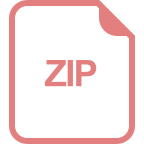
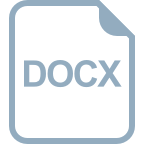
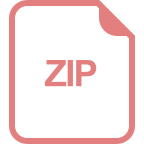
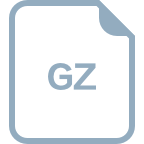
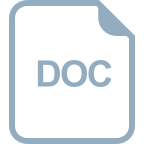
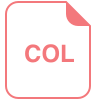
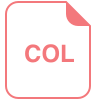
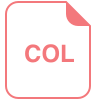
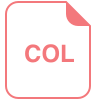
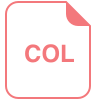
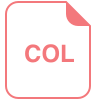
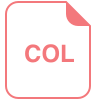
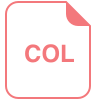
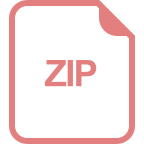