python实现present算法
时间: 2023-12-20 19:06:24 浏览: 28
PRESENT是一种流密码算法,它的加密过程中使用了代换和置换操作。下面是一个简单的Python实现:
```python
# S盒
SBox = [
0xc, 0x5, 0x6, 0xb, 0x9, 0x0, 0xa, 0xd,
0x3, 0xe, 0xf, 0x8, 0x4, 0x7, 0x1, 0x2
]
# P盒
PBox = [
0, 16, 32, 48, 1, 17, 33, 49,
2, 18, 34, 50, 3, 19, 35, 51,
4, 20, 36, 52, 5, 21, 37, 53,
6, 22, 38, 54, 7, 23, 39, 55,
8, 24, 40, 56, 9, 25, 41, 57,
10, 26, 42, 58, 11, 27, 43, 59,
12, 28, 44, 60, 13, 29, 45, 61,
14, 30, 46, 62, 15, 31, 47, 63
]
# 轮密钥
round_keys = [
0x0000000000000000, 0x0000000000000000, 0x0000000000000000, 0x0000000000000000,
0x0000000000000000, 0x0000000000000000, 0x0000000000000000, 0x0000000000000000,
0x0000000000000000, 0x0000000000000000, 0x0000000000000000, 0x0000000000000000,
0x0000000000000000, 0x0000000000000000, 0x0000000000000000, 0x0000000000000000,
0x0000000000000000, 0x0000000000000000, 0x0000000000000000, 0x0000000000000000,
0x0000000000000000, 0x0000000000000000, 0x0000000000000000, 0x0000000000000000,
0x0000000000000000, 0x0000000000000000, 0x0000000000000000, 0x0000000000000000,
0x0000000000000000, 0x0000000000000000, 0x0000000000000000, 0x0000000000000000,
0x0000000000000000, 0x0000000000000000, 0x0000000000000000, 0x0000000000000000,
0x0000000000000000, 0x0000000000000000, 0x0000000000000000, 0x0000000000000000,
0x0000000000000000, 0x0000000000000000, 0x0000000000000000, 0x0000000000000000,
0x0000000000000000, 0x0000000000000000, 0x0000000000000000, 0x0000000000000000,
0x0000000000000000, 0x0000000000000000, 0x0000000000000000, 0x0000000000000000,
0x0000000000000000, 0x0000000000000000, 0x0000000000000000, 0x0000000000000000,
0x0000000000000000, 0x0000000000000000, 0x0000000000000000, 0x0000000000000000,
0x0000000000000000, 0x0000000000000000, 0x0000000000000000, 0x0000000000000000,
0x0000000000000000
]
# 轮数
rounds = 31
# 加密函数
def encrypt(plaintext):
# 分组
state = [0] * 64
for i in range(8):
for j in range(8):
state[i*8+j] = (plaintext >> (i*8+j)*8) & 0xff
# 初始轮密钥加
for i in range(64):
state[i] ^= (round_keys[0] >> i) & 0x1
# 轮函数
for r in range(rounds):
# S盒代换
for i in range(64):
state[i] = SBox[state[i]]
# P盒置换
temp = [0] * 64
for i in range(64):
temp[PBox[i]] = state[i]
state = temp
# 轮密钥加
for i in range(64):
state[i] ^= (round_keys[r+1] >> i) & 0x1
# 输出密文
ciphertext = 0
for i in range(8):
ciphertext |= state[i*8:(i+1)*8][::-1] << i*8
return ciphertext
# 初始化轮密钥
def init_round_keys(key):
for i in range(rounds+1):
round_keys[i] = key
key = (key << 61) | (key >> 3)
key = ((SBox[(key >> 60) & 0xf] << 60) | (key << 4)) & 0xffffffffffffffff
# 测试
key = 0x000102030405060708090a0b0c0d0e0f
plaintext = 0x0123456789abcdef
init_round_keys(key)
ciphertext = encrypt(plaintext)
print('Plaintext:', hex(plaintext))
print('Ciphertext:', hex(ciphertext))
```
注意,在PRESENT算法中,每个字节的高位在前,低位在后。因此,我们需要做一些字节序的转换。此外,PRESENT算法的轮数和轮密钥的生成方式也是固定的。
相关推荐
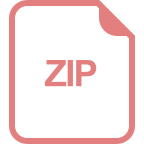
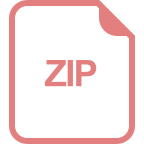
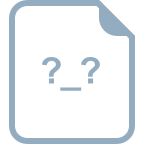










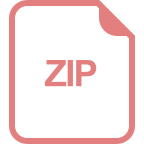
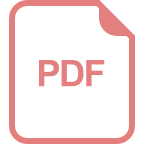
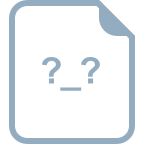
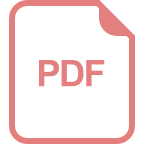