如何本地C# 通过APIpost 与网页通信
时间: 2024-10-04 17:00:49 浏览: 22
在C#中,要通过API发送POST请求与网页通信,你可以使用HttpClient类,这是一个非常常用的标准库工具。以下是简单的步骤:
1. 引入命名空间:
```csharp
using System.Net.Http;
using System.Text;
```
2. 创建HttpClient实例:
```csharp
HttpClient client = new HttpClient();
```
3. 准备POST数据(如果有需要的话,可以序列化到字符串形式,例如Json或FormUrlEncodedContent):
```csharp
string content = "your_data";
var formContent = new FormUrlEncodedContent(new[] { new KeyValuePair<string, string>("key", "value") });
```
或者如果是JSON格式的数据,可以这样做:
```csharp
string jsonContent = "{\"key\":\"value\"}";
var jsonContentString = JsonConvert.SerializeObject(jsonContent);
var jsonContent = new StringContent(jsonContentString, Encoding.UTF8, "application/json");
```
4. 发送POST请求:
```csharp
HttpResponseMessage response = await client.PostAsync("http://api.example.com/endpoint", formContent); // 或者jsonContent
```
5. 检查响应并处理结果:
```csharp
if (response.IsSuccessStatusCode)
{
string responseBody = await response.Content.ReadAsStringAsync();
Console.WriteLine(responseBody);
}
else
{
Console.WriteLine($"Error: {response.StatusCode}");
}
```
6. 可能还需要关闭连接:
```csharp
await client.Dispose(); // 当不再需要HttpClient时释放资源
```
阅读全文
相关推荐
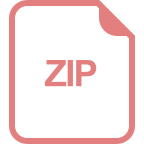
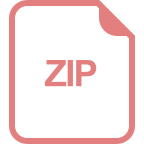
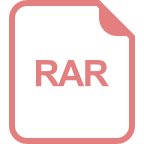

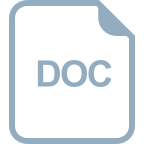
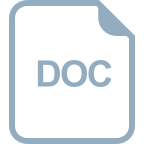
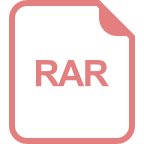
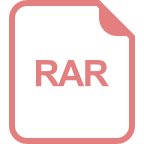
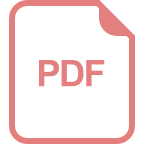
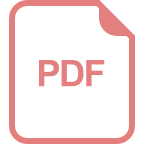
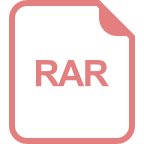
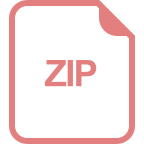
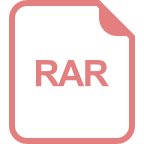
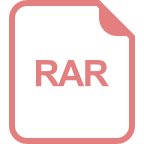
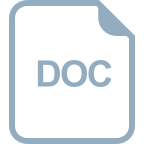
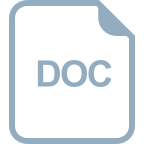
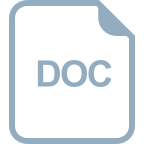
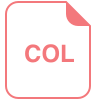
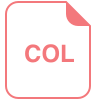