python向量加减乘除
时间: 2023-08-06 20:25:45 浏览: 136
在 Python 中,可以使用 NumPy 库来进行向量的加减乘除操作。假设有两个向量 a 和 b,可以通过以下方式进行操作:
1. 向量加法:a + b
2. 向量减法:a - b
3. 向量数乘:k * a,其中 k 为标量
4. 向量点乘:np.dot(a, b),其中 np 为导入的 NumPy 库
需要注意的是,进行向量加减法时,要确保两个向量的维度相同;进行向量点乘时,要确保两个向量的长度相同。
相关问题
python实现向量加减乘除
在 Python 中,可以使用 NumPy 库来进行向量的加减乘除操作。以下是示例代码:
```python
import numpy as np
# 定义两个向量
a = np.array([1, 2, 3])
b = np.array([4, 5, 6])
# 向量加法
c = a + b
print("向量加法结果:", c)
# 向量减法
d = a - b
print("向量减法结果:", d)
# 向量数乘
k = 2
e = k * a
print("向量数乘结果:", e)
# 向量点乘
f = np.dot(a, b)
print("向量点乘结果:", f)
```
输出结果为:
```
向量加法结果: [5 7 9]
向量减法结果: [-3 -3 -3]
向量数乘结果: [2 4 6]
向量点乘结果: 32
```
需要注意的是,在进行向量加减法时,要确保两个向量的维度相同;在进行向量点乘时,要确保两个向量的长度相同。
python用类实现向量加减乘除
可以通过定义一个向量类来实现向量的加减乘除操作。以下是示例代码:
```python
import numpy as np
class Vector:
def __init__(self, data):
self.data = np.array(data)
def __add__(self, other):
if isinstance(other, Vector):
return Vector(self.data + other.data)
else:
raise TypeError("Unsupported operand type for +")
def __sub__(self, other):
if isinstance(other, Vector):
return Vector(self.data - other.data)
else:
raise TypeError("Unsupported operand type for -")
def __mul__(self, other):
if isinstance(other, (int, float)):
return Vector(self.data * other)
elif isinstance(other, Vector):
return np.dot(self.data, other.data)
else:
raise TypeError("Unsupported operand type for *")
def __truediv__(self, other):
if isinstance(other, (int, float)):
return Vector(self.data / other)
else:
raise TypeError("Unsupported operand type for /")
def __repr__(self):
return f"Vector({self.data})"
```
在这个向量类中,定义了向量加减乘除的运算符重载方法。可以使用 Vector([1, 2, 3]) 来创建一个向量对象,然后调用向量的加减乘除方法来进行相应的操作。示例代码如下:
```python
# 定义两个向量
a = Vector([1, 2, 3])
b = Vector([4, 5, 6])
# 向量加法
c = a + b
print("向量加法结果:", c)
# 向量减法
d = a - b
print("向量减法结果:", d)
# 向量数乘
k = 2
e = k * a
print("向量数乘结果:", e)
# 向量点乘
f = a * b
print("向量点乘结果:", f)
```
输出结果为:
```
向量加法结果: Vector([5 7 9])
向量减法结果: Vector([-3 -3 -3])
向量数乘结果: Vector([2 4 6])
向量点乘结果: 32
```
需要注意的是,在进行向量加减法时,要确保两个向量的维度相同;在进行向量点乘时,要确保两个向量的长度相同。
阅读全文
相关推荐
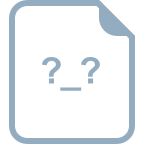
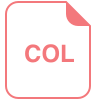





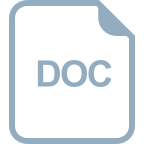
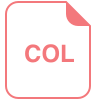




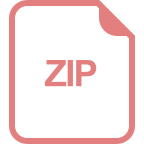