int i=1; while (rs.next()) { System.out.println(i++); String json = "{" + "\"cityName\":" + "\"" + rs.getString(1) + "\"" + "," + "\"value\":" + "\"" + rs.getInt(2) + "\"" + "}"; result = result + json + ","; } result = "[" + result.substring(0, result.length() - 1) + "]";
时间: 2023-07-12 18:03:46 浏览: 71
这段代码看起来是Java代码。它的作用是从数据库中查询出城市名称和对应的数值,然后将它们拼接成JSON字符串的形式,最后将这些JSON字符串拼接成一个JSON数组返回。其中,使用了ResultSet对象的next()方法遍历数据库查询结果,使用了字符串拼接来构造JSON字符串,并使用了字符串截取来去掉最后一个逗号并在两端添加方括号构成JSON数组。
相关问题
ResultSet转为JSON
ResultSet 是 Java 中的一个接口,用于表示数据库查询结果集。如果要将 ResultSet 转为 JSON 格式,可以使用以下步骤:
1. 将 ResultSet 中的数据转为 Java 对象,如 List<Map<String, Object>>。
2. 使用 JSON 库将 Java 对象转为 JSON 格式,如 Gson 或 Jackson。
下面是使用 Gson 将 ResultSet 转为 JSON 的示例代码:
```java
import com.google.gson.Gson;
import java.sql.*;
import java.util.*;
public class ResultSetToJson {
public static void main(String[] args) throws SQLException {
String url = "jdbc:mysql://localhost:3306/mydatabase";
String user = "root";
String password = "mypassword";
try (Connection conn = DriverManager.getConnection(url, user, password);
Statement stmt = conn.createStatement();
ResultSet rs = stmt.executeQuery("SELECT * FROM users")) {
List<Map<String, Object>> resultList = new ArrayList<>();
ResultSetMetaData metaData = rs.getMetaData();
int columnCount = metaData.getColumnCount();
while (rs.next()) {
Map<String, Object> row = new HashMap<>();
for (int i = 1; i <= columnCount; i++) {
row.put(metaData.getColumnLabel(i), rs.getObject(i));
}
resultList.add(row);
}
Gson gson = new Gson();
String json = gson.toJson(resultList);
System.out.println(json);
}
}
}
```
在上面的示例代码中,使用了 com.google.gson 包中的 Gson 类将 List<Map<String, Object>> 对象转为 JSON 格式。可以根据需要修改代码中的数据库连接信息和查询语句。
sql json 查询 jsonObject
以下是将SQL查询结果封装成JSON对象的示例代码:
```java
import java.sql.*;
import org.json.*;
public class SqlToJson {
public static void main(String[] args) {
String url = "jdbc:mysql://localhost:3306/test";
String user = "root";
String password = "123456";
String sql = "SELECT id, username, password, sex FROM user WHERE id = ?";
int id = 1;
try {
Class.forName("com.mysql.jdbc.Driver");
Connection conn = DriverManager.getConnection(url, user, password);
PreparedStatement ps = conn.prepareStatement(sql);
ps.setInt(1, id);
ResultSet rs = ps.executeQuery();
JSONObject json = new JSONObject();
while (rs.next()) {
json.put("id", rs.getInt("id"));
json.put("username", rs.getString("username"));
json.put("password", rs.getString("password"));
json.put("sex", rs.getString("sex"));
}
System.out.println(json.toString());
conn.close();
} catch (Exception e) {
e.printStackTrace();
}
}
}
```
这个示例代码使用了Java语言和MySQL数据库。首先,我们需要导入Java的SQL包和JSON包。然后,我们定义了数据库的连接信息、SQL语句和查询参数。接着,我们使用JDBC连接数据库,并执行预处理的SQL语句。在结果集中,我们将每一行的数据封装成一个JSON对象。最后,我们将JSON对象转换成字符串并输出。
阅读全文
相关推荐
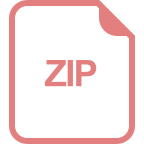
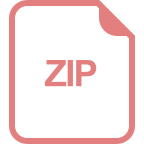
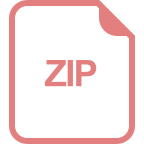
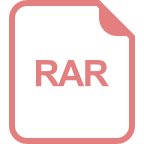
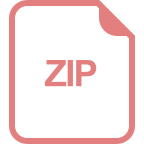
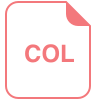
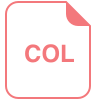
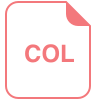
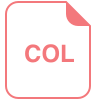
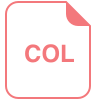
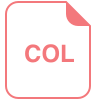
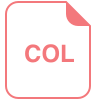
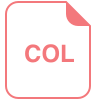
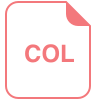


