c语言实现2 随机抽取一个单词,界面显示该单词的首尾字母以及中间空缺位符,对应的释义,缺失的部分由玩家输入,显示游戏剩余的错误次数,显示出题目的同时开始计时 3 玩家输入完成后,判断其是否正确,当答题剩余3秒时进行提示 4 如果回答正确,则玩家分数加1;如果超时或回答错误,判断为错误,错误剩余次数减1 5 不断重复前面的过程,当剩余错误次数为零时,游戏结束
时间: 2023-11-05 16:04:14 浏览: 45
以下是一个简单的实现:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <time.h>
#define WORD_COUNT 5 // 单词数量
#define MAX_WORD_LEN 20 // 最大单词长度
#define MAX_WRONG_GUESSES 5 // 最大错误次数
#define HIDDEN_CHAR '-' // 隐藏字符
const char *WORDS[WORD_COUNT] = {"apple", "banana", "orange", "grape", "peach"};
const char *DEFINITIONS[WORD_COUNT] = {
"a round fruit with red, green, or yellow skin and a white center",
"a long curved fruit with a yellow skin",
"a round fruit with an orange skin and a sweet juice",
"a small round fruit that is usually purple or green",
"a fruit with a fuzzy yellow or pink skin and a juicy yellow center"
};
int main()
{
srand(time(NULL)); // 初始化随机数生成器
int score = 0; // 玩家得分
int wrong_guesses = 0; // 错误次数
clock_t start_time; // 开始时间
clock_t end_time; // 结束时间
int word_index = rand() % WORD_COUNT; // 随机选择一个单词
const char *word = WORDS[word_index];
const char *definition = DEFINITIONS[word_index];
int len = strlen(word);
char hidden_word[MAX_WORD_LEN + 1];
memset(hidden_word, HIDDEN_CHAR, sizeof(hidden_word));
hidden_word[0] = word[0];
hidden_word[len - 1] = word[len - 1];
printf("Word: %s\n", hidden_word);
printf("Definition: %s\n", definition);
start_time = clock(); // 记录开始时间
while (wrong_guesses < MAX_WRONG_GUESSES)
{
printf("Guess a letter or the whole word: ");
char guess[MAX_WORD_LEN + 1];
scanf("%s", guess);
if (strcmp(guess, word) == 0) // 猜对了整个单词
{
printf("Correct!\n");
score++;
break;
}
else if (strlen(guess) == 1) // 猜对了一个字母
{
int found = 0;
for (int i = 1; i < len - 1; i++)
{
if (word[i] == guess[0])
{
hidden_word[i] = guess[0];
found = 1;
}
}
if (found)
{
printf("Correct!\n");
printf("Word: %s\n", hidden_word);
}
else
{
printf("Wrong!\n");
wrong_guesses++;
printf("You have %d wrong guesses left.\n", MAX_WRONG_GUESSES - wrong_guesses);
}
}
else // 猜错了整个单词
{
printf("Wrong!\n");
wrong_guesses++;
printf("You have %d wrong guesses left.\n", MAX_WRONG_GUESSES - wrong_guesses);
}
if (strcmp(hidden_word, word) == 0) // 猜对了整个单词
{
printf("Correct!\n");
score++;
break;
}
end_time = clock(); // 记录结束时间
double elapsed_time = (double)(end_time - start_time) / CLOCKS_PER_SEC;
if (elapsed_time >= 57) // 剩余3秒
{
printf("Time is running out!\n");
}
}
printf("Game over! Your score is %d.\n", score);
return 0;
}
```
该程序随机选择一个单词,并显示该单词的首尾字母以及中间的空缺字符。玩家需要猜测单词中的字母或整个单词,并在限定的错误次数内猜对。如果猜对了一个字母,程序会显示出现的位置;如果猜对了整个单词,程序会给出正确提示并加分;如果超时或猜错了整个单词,程序会减少错误次数。当错误次数达到最大值时,游戏结束,显示玩家得分。
相关推荐
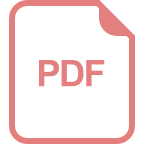
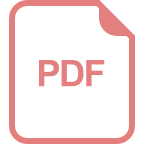














