能用c++写费诺编码的文本压缩
时间: 2024-01-22 12:20:51 浏览: 27
当然可以,费诺编码是一种无损压缩算法,可以将文本数据压缩为更小的存储空间。以下是一个简单的C++实现:
```cpp
#include <iostream>
#include <string>
#include <map>
#include <vector>
#include <algorithm>
struct Node {
char ch;
int freq;
Node* left;
Node* right;
Node(char ch, int freq) : ch(ch), freq(freq), left(nullptr), right(nullptr) {}
};
void build_frequency_table(const std::string& input, std::map<char, int>& frequency_table) {
for (char ch : input) {
frequency_table[ch]++;
}
}
Node* build_huffman_tree(std::map<char, int>& frequency_table) {
std::vector<Node*> nodes;
for (auto it = frequency_table.begin(); it != frequency_table.end(); ++it) {
nodes.push_back(new Node(it->first, it->second));
}
while (nodes.size() > 1) {
std::sort(nodes.begin(), nodes.end(), [](Node* node1, Node* node2) { return node1->freq > node2->freq; });
Node* left = nodes.back();
nodes.pop_back();
Node* right = nodes.back();
nodes.pop_back();
Node* parent = new Node('\0', left->freq + right->freq);
parent->left = left;
parent->right = right;
nodes.push_back(parent);
}
return nodes.front();
}
void build_encoding_table(Node* root, std::string& code, std::map<char, std::string>& encoding_table) {
if (!root) {
return;
}
if (root->ch != '\0') {
encoding_table[root->ch] = code;
}
code.push_back('0');
build_encoding_table(root->left, code, encoding_table);
code.pop_back();
code.push_back('1');
build_encoding_table(root->right, code, encoding_table);
code.pop_back();
}
std::string encode(const std::string& input, std::map<char, std::string>& encoding_table) {
std::string output;
for (char ch : input) {
output += encoding_table[ch];
}
return output;
}
int main() {
std::string input = "hello world";
std::map<char, int> frequency_table;
build_frequency_table(input, frequency_table);
Node* root = build_huffman_tree(frequency_table);
std::string code;
std::map<char, std::string> encoding_table;
build_encoding_table(root, code, encoding_table);
std::string encoded = encode(input, encoding_table);
std::cout << encoded << std::endl;
return 0;
}
```
这个实现包含了几个步骤:
1. 构建字符频率表:对输入字符串进行遍历,统计每个字符出现的次数;
2. 构建哈夫曼树:将频率表中的每个字符转换为一个节点,然后通过合并节点的方式构建哈夫曼树;
3. 构建编码表:通过遍历哈夫曼树,生成每个字符的编码表;
4. 压缩数据:将输入字符串中的每个字符使用编码表进行替换,生成压缩后的字符串。
这个实现只是一个简单的例子,没有进行错误处理和优化,但可以作为费诺编码的入门示例。
相关推荐
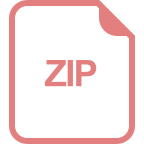
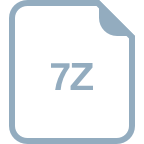
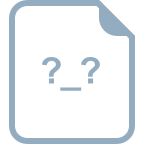














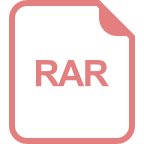