java实现mysql数据库表迁移代码
时间: 2023-09-15 08:18:03 浏览: 48
实现 mysql 数据库表迁移的代码可以使用 Java 的 JDBC API 来进行操作。下面是一个简单的示例代码:
```
import java.sql.*;
public class TableMigration {
public static void main(String[] args) {
Connection sourceConn = null;
Connection targetConn = null;
try {
// 配置源数据库连接
sourceConn = DriverManager.getConnection("jdbc:mysql://localhost:3306/source_db?useSSL=false", "root", "password");
// 配置目标数据库连接
targetConn = DriverManager.getConnection("jdbc:mysql://localhost:3306/target_db?useSSL=false", "root", "password");
// 获取源数据库表列表
ResultSet rs = sourceConn.getMetaData().getTables(null, null, "%", new String[]{"TABLE"});
while (rs.next()) {
String tableName = rs.getString("TABLE_NAME");
System.out.println("Migrating table " + tableName + "...");
migrateTable(sourceConn, targetConn, tableName);
}
System.out.println("All tables migrated successfully!");
} catch (SQLException ex) {
ex.printStackTrace();
} finally {
// 关闭数据库连接
if (sourceConn != null) {
try {
sourceConn.close();
} catch (SQLException ex) {
ex.printStackTrace();
}
}
if (targetConn != null) {
try {
targetConn.close();
} catch (SQLException ex) {
ex.printStackTrace();
}
}
}
}
private static void migrateTable(Connection sourceConn, Connection targetConn, String tableName) {
try {
// 查询源数据库表的数据
Statement sourceStmt = sourceConn.createStatement();
ResultSet sourceRs = sourceStmt.executeQuery("SELECT * FROM " + tableName);
// 获取源数据库表的列信息
ResultSetMetaData sourceMeta = sourceRs.getMetaData();
int columnCount = sourceMeta.getColumnCount();
StringBuilder columns = new StringBuilder();
for (int i = 1; i <= columnCount; i++) {
String columnName = sourceMeta.getColumnName(i);
String columnType = sourceMeta.getColumnTypeName(i);
columns.append(columnName).append(" ").append(columnType).append(",");
}
columns.deleteCharAt(columns.length() - 1);
// 在目标数据库中创建表
Statement targetStmt = targetConn.createStatement();
targetStmt.execute("CREATE TABLE " + tableName + " (" + columns + ")");
// 循环插入数据到目标数据库表中
while (sourceRs.next()) {
StringBuilder values = new StringBuilder();
for (int i = 1; i <= columnCount; i++) {
String value = sourceRs.getString(i);
values.append("'").append(value).append("',");
}
values.deleteCharAt(values.length() - 1);
targetStmt.executeUpdate("INSERT INTO " + tableName + " VALUES (" + values + ")");
}
// 关闭资源
sourceRs.close();
sourceStmt.close();
targetStmt.close();
} catch (SQLException ex) {
ex.printStackTrace();
}
}
}
```
注:以上代码仅供参考,具体需要根据不同的数据库结构进行调整。
相关推荐
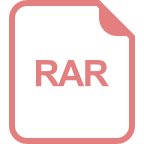














