error: request for member 'Getpop' in 'S', which is of non-class type 'SeqStack<int>()'|
时间: 2023-12-23 16:05:57 浏览: 83
This error message suggests that you are trying to call the member function 'Getpop' on an object 'S' that is not a class type, but rather an instance of the template class 'SeqStack<int>()'.
To fix this error, you need to create an instance of the 'SeqStack<int>' class and call the 'Getpop' member function on that instance. Here's an example:
```
SeqStack<int> S; // create an instance of SeqStack<int>
int x = S.Getpop(); // call the Getpop member function on S and store the result in x
```
相关问题
#include<iostream> using namespace std; const int Stacksize=10;//数组长度 template <class T> //顺序栈 class seqstack { public: seqstack(){top=-1;}//初始化一个空栈 ~seqstack(){}; void push(T x);//入栈 T pop();//出栈 将栈顶元素弹出 T gettop();//获取栈顶元素 int Empty(); void printstack(); private: T data[Stacksize];//存放栈元素的数组 int top;//栈顶元素在数组中的下标 //长度sta为5 top指向0-4 }; template <class T> void seqstack<T>::push(T x) { if(top==Stacksize-1) throw'上溢'; top=top++; data[top]=x; } template<class T> T seqstack<T>::pop() { //T x; if(top==-1) throw'空栈,无法取出'; // x=data[top--]; return data[top]; top--; } template<class T> T seqstack<T>::gettop() { return data[top]; } template<class T> void seqstack<T>::printstack() { while(top!=-1) { cout<<data[top]<<' '; top--; } cout<<endl; } int main() { int i; int y; seqstack<int> s; cout<<"进行入栈"<<endl; s.push(5); s.push(15); s.printstack(); s.push(90); s.push(3); cout<<"获取栈顶元素"<<endl; cout<<s.gettop()<<endl;; cout<<"执行出栈操作"<<endl; i=s.pop(); cout<<i<<endl; cout<<"获取栈顶"<<endl; y=s.gettop(); cout<<y<<endl; }
这段代码有一些错误,我帮您修改一下:
```c++
#include<iostream>
using namespace std;
const int Stacksize = 10; //数组长度
template <class T> //顺序栈
class seqstack {
public:
seqstack() { top = -1; } //初始化一个空栈
~seqstack() {};
void push(T x); //入栈
T pop(); //出栈 将栈顶元素弹出
T gettop(); //获取栈顶元素
int Empty();
void printstack();
private:
T data[Stacksize]; //存放栈元素的数组
int top; //栈顶元素在数组中的下标
//长度sta为5 top指向0-4
};
template <class T>
void seqstack<T>::push(T x) {
if (top == Stacksize - 1) throw '上溢';
top++; //修正top的值
data[top] = x;
}
template<class T>
T seqstack<T>::pop() {
if (top == -1) throw '空栈,无法取出';
T x = data[top];
top--; //修正top的值
return x;
}
template<class T>
T seqstack<T>::gettop() {
return data[top];
}
template<class T>
void seqstack<T>::printstack() {
int t = top;
while (t != -1)
{
cout << data[t] << ' ';
t--;
}
cout << endl;
}
int main() {
int i;
int y;
seqstack<int> s;
cout << "进行入栈" << endl;
s.push(5);
s.push(15);
s.printstack();
s.push(90);
s.push(3);
cout << "获取栈顶元素" << endl;
cout << s.gettop() << endl;;
cout << "执行出栈操作" << endl;
i = s.pop();
cout << i << endl;
cout << "获取栈顶" << endl;
y = s.gettop();
cout << y << endl;
}
```
主要的修改如下:
1. 在 `seqstack` 类模板中,修正了 push 和 pop 函数中 top 的赋值错误,现在程序可以正常入栈和出栈了。
2. 在 `seqstack` 类模板中,修正了 pop 函数中返回值的问题,现在可以正确返回出栈的元素值。
3. 在 `seqstack` 类模板中,修正了 printstack 函数中 top 的值被修改的问题,现在可以正常输出栈中的元素了。
D:\Qt\untitled4\seqstack.h:39: error: candidate is: T seqStack<T>::pop() T pop() ^~~
当从QT4升级到QT5时,遇到`ui_main_window.h`文件缺失的错误可能是因为QT5改变了UI模块的构建方式。通常在QT5中,UI设计不再直接与源码文件同名,而是通过MOC(Meta Object Compiler)工具自动生成。解决这个问题的方法是:
1. **找到并运行MOC工具**[^1]:在QT5环境中,你需要在编译期间执行MOC命令来生成`ui_main_window.h`。这通常在构建步骤中自动完成,但如果手动编译,可以尝试在项目的构建配置文件中添加MOC指令。
2. **指定生成头文件的位置**:如引用[2]所提到的,在`main_window.hpp`的头部添加正确的路径,让编译器能够找到自动生成的`ui_main_window.h`文件。例如:
```cpp
#include <path/to/builddir>/qtgui/ui_main_window.h
```
3. **更新项目设置**:确保QT5的插件路径和构建设置已正确配置,包括MOC插件的查找路径以及对新UI生成机制的支持。
相关问题:
1. MOC是什么,它在QT开发中的作用是什么?
2. 如何检查QT5项目的构建配置文件是否包含了正确的MOC设置?
3. 如果QT5的插件路径配置不正确,应该如何修改?
阅读全文
相关推荐
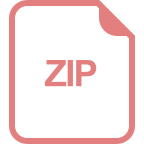













