import jieba import wordcloud import imageio.v2 as imageio #第三方库需要安装 f = open("规划.txt", "r", encoding="utf-8") t = f.read() f.close() ls = jieba.lcut(t) txt = " ".join(ls) pic=imageio.imread("广东省.png") w = wordcloud.WordCloud(\ width = 1000, height = 700,\ background_color = "white", font_path = "msyh.ttf", mask = pic)#引用词云图片样式,字体文件要和程序放在同一个文件夹里 w.generate(txt) w.to_file("grwordcloud1.png")
时间: 2023-11-02 19:04:55 浏览: 101
='center',va='center',fontsize=10)
# 设置坐标轴范围和刻度
ax.set_xlim(-1这是一个使用Python编写的生成中文词云的代码示例,具体实现步骤如下:
1. 导入需要的Python库,包括jieba、wordcloud和imageio。
2. 打开文本文件“规划.txt,28)
ax.set_ylim(-1,1)
plt.xticks([])
plt.yticks([])
plt.show()
# 调用函数”,并读取其中的内容。
3. 使用jieba对文本进行分词,得到分词结果ls。
4.绘制地铁线路图
draw_subway_map()
```
您可以根据需要调整图形的颜色、字 将分词结果ls中的单词用空格连接成一个字符串txt。
5. 使用imageio读取词云图片样体、线条粗细等参数,以创建更美观的地铁线路图。
相关问题
修改代码import jieba import numpy as np import matplotlib.pyplot as plt text = open('comment.txt', encoding='utf-8').read() from PIL import Image, ImageDraw, ImageFont from wordcloud import WordCloud text = ' '.join(jieba.cut(text)) # 生成对象 mask = np.array(Image.open("computer.jpg")) wc = WordCloud(ImageFont.truetype("arial.ttf")).generate(text) # 显示词云 # plt.imshow(wc, interpolation = 'bilinear') # plt.axis("off") # plt.show() # 保存文件 wc.to_file('rs_computer.png')
这段代码的问题在于 `WordCloud` 类的实例化时,使用了错误的参数格式。`WordCloud` 类的构造函数需要传入一个字典类型的参数,用于指定词云生成时的各种参数。而在这段代码中,只传入了一个 `ImageFont.truetype("arial.ttf")` 参数,这是错误的。
下面是修改后的代码,已经添加了 `WordCloud` 类的参数字典:
```python
import jieba
import numpy as np
import matplotlib.pyplot as plt
from PIL import Image, ImageDraw, ImageFont
from wordcloud import WordCloud
text = open('comment.txt', encoding='utf-8').read()
text = ' '.join(jieba.cut(text))
# 生成对象
mask = np.array(Image.open("computer.jpg"))
wc = WordCloud(
font_path=ImageFont.truetype("arial.ttf"),
mask=mask,
background_color="white",
max_words=2000,
max_font_size=100,
width=1600,
height=800,
random_state=42
).generate(text)
# 显示词云
# plt.imshow(wc, interpolation='bilinear')
# plt.axis("off")
# plt.show()
# 保存文件
wc.to_file('rs_computer.png')
```
在这个例子中,我们使用了 `WordCloud` 类的参数字典,指定了生成词云时的各种参数,例如字体文件路径、遮罩图像、背景颜色、最大单词数、最大字体大小、词云图像宽度和高度等。这样就可以正确地生成和保存词云图像了。
import jieba import wordcloud import imageio mask = imageio.imread('C:/Users/Febird/Desktop/1.png') #设定一个词云背景 with open('C:/Users/Febird/Desktop/傲世.txt', encoding='utf-8') as f: t = f.read() # 打开需要制作词云图的文件 ls = jieba.lcut(t) txt = " ".join(ls) # 将文章中的词组提出来 stopwords_file = open('C:/Users/Febird/Desktop/stopWord.txt', 'r', encoding='utf-8') stopwords = [(words.strip()) for words in stopwords_file.readlines()] w = wordcloud.WordCloud(width=4000, height=4000, stopwords=stopwords, font_path="msyh.ttc", colormap='hot', background_color='pink', mask=mask) # 定义一个词云 w.generate(txt) #生成词云 w.to_file(r'C:/Users/Febird/Desktop/test_3.png') #将词组变量txt导入词云对象w中并保存 添加代码 完成排名前五十个词的词云
在代码中添加以下部分可以完成排名前五十个词的词云:
```
from collections import Counter
# 统计词频
words_count = Counter(ls)
# 获取词频前50的词及其频次
top50_words = words_count.most_common(50)
# 将排名前50的词转换为一个字符串
top50_str = ""
for word, count in top50_words:
top50_str += (word + " ") * count
# 生成词云
w.generate(top50_str)
```
完整代码如下:
```
import jieba
import wordcloud
import imageio
from collections import Counter
mask = imageio.imread('C:/Users/Febird/Desktop/1.png')
with open('C:/Users/Febird/Desktop/傲世.txt', encoding='utf-8') as f:
t = f.read()
ls = jieba.lcut(t)
txt = " ".join(ls)
stopwords_file = open('C:/Users/Febird/Desktop/stopWord.txt', 'r', encoding='utf-8')
stopwords = [(words.strip()) for words in stopwords_file.readlines()]
w = wordcloud.WordCloud(width=4000, height=4000,
stopwords=stopwords,
font_path="msyh.ttc",
colormap='hot',
background_color='pink',
mask=mask)
# 统计词频
words_count = Counter(ls)
# 获取词频前50的词及其频次
top50_words = words_count.most_common(50)
# 将排名前50的词转换为一个字符串
top50_str = ""
for word, count in top50_words:
top50_str += (word + " ") * count
# 生成词云
w.generate(top50_str)
w.to_file(r'C:/Users/Febird/Desktop/test_3.png')
```
阅读全文
相关推荐
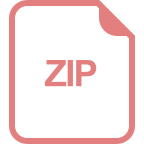
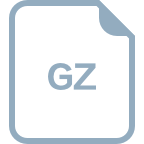













