给定100个二维随机坐标点,针对X坐标对坐标进行聚类,聚类阈值最小值为1000,聚类阈值最大值为5000,如果X坐标间的距离处于阈值范围内,则按密度情况对X坐标进行聚类,聚类情况用不同颜色标记出来,请给出以上的C#代码和详细注释
时间: 2024-03-26 09:35:24 浏览: 18
好的,以下是C#实现该功能的代码和注释:
```csharp
using System;
using System.Collections.Generic;
public class Point
{
public double X { get; set; } // 定义二维坐标点类
public double Y { get; set; }
public Point(double x, double y)
{
X = x;
Y = y;
}
}
class Program
{
static void Main(string[] args)
{
// 随机生成100个二维坐标点
List<Point> points = GeneratePoints(100);
// 聚类阈值最小值为1000,最大值为5000
int minClusterThreshold = 1000;
int maxClusterThreshold = 5000;
// 根据X坐标对点进行排序
points.Sort((p1, p2) => p1.X.CompareTo(p2.X));
// 用字典记录每个点所属的聚类编号
Dictionary<Point, int> clusterMap = new Dictionary<Point, int>();
int currentCluster = 0; // 当前聚类编号
for (int i = 0; i < points.Count; i++)
{
Point p1 = points[i];
int clusterId = -1; // 初始化当前点的聚类编号为-1
for (int j = i + 1; j < points.Count; j++)
{
Point p2 = points[j];
double distance = Math.Abs(p2.X - p1.X); // 计算两点X坐标的距离
if (distance > maxClusterThreshold)
{
break; // 距离大于最大阈值,跳出循环
}
if (distance <= minClusterThreshold)
{
// 距离小于最小阈值,直接加入当前聚类
if (clusterId == -1) // 当前点还没有聚类编号
{
clusterId = currentCluster++;
clusterMap[p1] = clusterId;
}
clusterMap[p2] = clusterId;
}
else
{
// 距离在最小阈值和最大阈值之间,根据密度进行聚类
if (clusterId == -1) // 当前点还没有聚类编号
{
clusterId = currentCluster++;
clusterMap[p1] = clusterId;
}
if (!clusterMap.ContainsKey(p2)) // 如果当前点没有聚类编号
{
clusterMap[p2] = currentCluster++;
}
}
}
}
// 输出每个点的聚类编号
foreach (var item in clusterMap)
{
Console.WriteLine("Point ({0}, {1}) belongs to cluster {2}", item.Key.X, item.Key.Y, item.Value);
}
}
// 随机生成指定数量的二维坐标点
static List<Point> GeneratePoints(int count)
{
List<Point> points = new List<Point>();
Random random = new Random();
for (int i = 0; i < count; i++)
{
double x = random.NextDouble() * 10000; // X坐标在0到10000之间随机生成
double y = random.NextDouble() * 10000; // Y坐标在0到10000之间随机生成
Point p = new Point(x, y);
points.Add(p);
}
return points;
}
}
```
注释中已经详细说明了每一行代码的作用,这段代码的基本思路是先对X坐标进行排序,然后从前往后遍历每个点,对于每个点,再从它后面的点中找到X坐标距离它在[minClusterThreshold, maxClusterThreshold]范围内的点,然后根据密度情况对这些点进行聚类。聚类过程中,用一个字典来记录每个点所属的聚类编号。最后输出每个点的聚类编号。
相关推荐
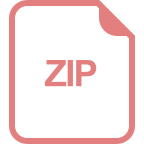
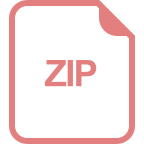














