写一个c++程序将任意多边形的顶点坐标绕形心按逆时针排序,并输出排序结果
时间: 2023-02-12 11:21:27 浏览: 115
```c
#include <stdio.h>
#include <math.h>
struct Point {
double x, y;
} polygon[100];
int n;
int compare(const void* a, const void* b) {
Point* p1 = (Point*)a;
Point* p2 = (Point*)b;
double angle1 = atan2(p1->y, p1->x);
double angle2 = atan2(p2->y, p2->x);
if (angle1 < angle2) {
return -1;
} else if (angle1 > angle2) {
return 1;
} else {
return 0;
}
}
int main() {
printf("请输入多边形顶点数量:");
scanf("%d", &n);
double x = 0, y = 0;
printf("请输入多边形顶点坐标:\n");
for (int i = 0; i < n; i++) {
scanf("%lf %lf", &polygon[i].x, &polygon[i].y);
x += polygon[i].x;
y += polygon[i].y;
}
// 求形心
Point centroid = {x / n, y / n};
for (int i = 0; i < n; i++) {
polygon[i].x -= centroid.x;
polygon[i].y -= centroid.y;
}
qsort(polygon, n, sizeof(Point), compare);
printf("排序后的顶点坐标:\n");
for (int i = 0; i < n; i++) {
printf("%.2lf %.2lf\n", polygon[i].x + centroid.x, polygon[i].y + centroid.y);
}
return 0;
}
```
程序中使用了`qsort`函数进行顶点坐标的排序,比较函数中使用了`atan2`函数来计算顶点坐标相对于形心的极角。程序首先计算出形心的坐标,然后将顶点坐标减去形心的坐标进行排序,最后再加上形心的坐标输出。
阅读全文
相关推荐
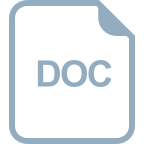
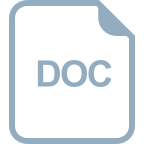
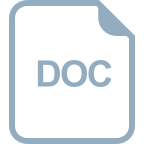
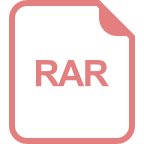
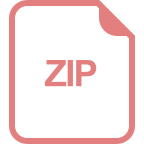
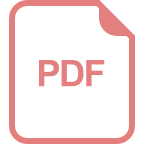
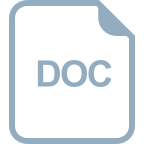
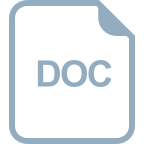









